继承
1. 继承的概念
- 继承是在现有类的基础上创建新类的一种手段,不需要知道现有类的实现
- 现有的类称为基类,创建的新类称为派生类
- 基类也称为父类,派生类也称为子类
- 父类派生了子类,子类继承于父类
- 子类可以在父类基础上添加新成员变量(属性)和新成员函数(方法),还可以重写父类成员函数的实现
- 继承表达的是子类和父类的”is-a”关系,不满足”is-a”关系的继承是不合理的
- 举例:学生是人,Student和Person就是继承关系
继承方式:
除了三大成员函数,子类继承了父类的全部成员变量和全部成员函数与
- 未被继承的父类的三大成员函数是:
- 1> 构造函数(包括拷贝构造函数)
- 2> 析构函数
- 3> 赋值运算符函数
- 示例代码:
```cpp
include
using namespace std;
class Person{ private: string name; bool gender; int age; public: Person(){ cout << “Person()” << endl; } Person(string name, bool gender, int age){ name = name; gender = gender; age = age; cout << “Person(string, bool, int)” << endl; } void show(){ cout << “本人名叫” << name << endl; cout << (gender == true ? “帅哥一枚” : “美女一枚”) << endl; cout << “年方” << age << “青春无敌”<< endl; } };
class Student : public Person{ };
class Teacher : protected Person{ };
class Coder : private Person{ };
class Kong{ };
int main(int argc, const char** argv){ cout << “sizeof(Person) : “ << sizeof(Person) << endl; cout << “sizeof(Student) : “ << sizeof(Student) << endl; cout << “sizeof(Teacher) : “ << sizeof(Teacher) << endl; cout << “sizeof(Coder) : “ << sizeof(Coder) << endl; cout << “sizeof(Kong) : “ << sizeof(Kong) << endl;
Student st;
st.show();
Teacher th;
//th.show(); // 无法调用show()
Coder cd;
//cd.show(); // 无法调用show()
return 0;
}
<a name="oG3Yv"></a>
## 3. 继承方式与访问权限
- 子类继承了父类的成员,但是并没有继承相应的访问权限,其从父类继承过来的成员的访问权限,受继承方式的影响。
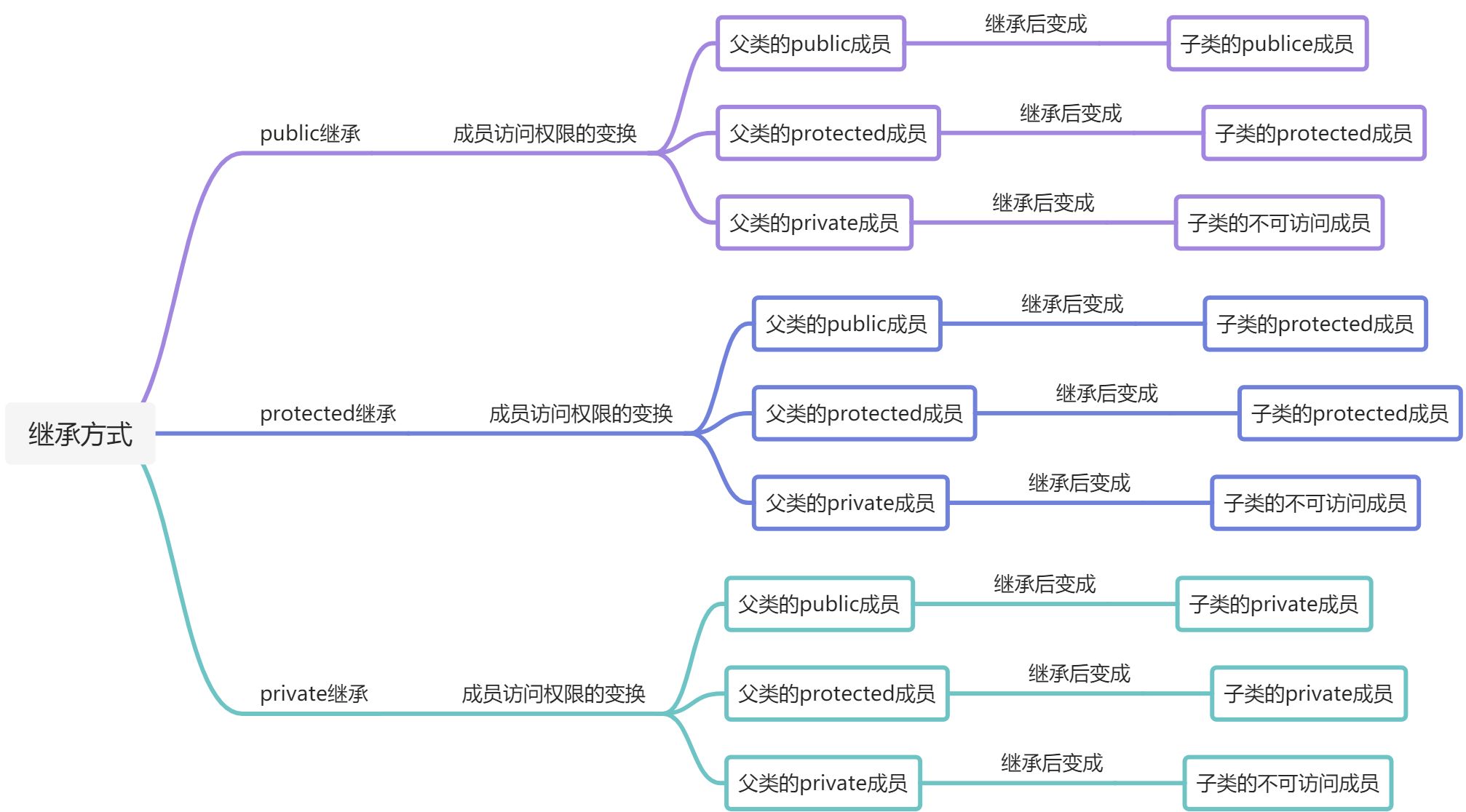
- 示例代码:
```cpp
using namespace std;
#include <iostream>
class Person{
private:
string name_;
bool gender_;
int age_;
public:
Person(){
cout << "Person()" << endl;
}
Person(string name, bool gender, int age){
name_ = name;
gender_ = gender;
age_ = age;
cout << "Person(string, bool, int)" << endl;
}
void show(){
cout << "本人名叫" << name_ << endl;
cout << (gender_ == true ? "帅哥一枚" : "美女一枚") << endl;
cout << "年方" << age_ << "青春无敌"<< endl;
}
protected:
void protectedFunc(){
cout << "protectedFunc()" << endl;
}
};
class Student : public Person{
public:
void testRight(){
protectedFunc(); // protected
//cout << name << endl; // error, 不可访问
}
};
class Teacher : protected Person{
};
class Coder : private Person{
};
int main(int argc, const char** argv){
Student st;
st.show();
//st.protectedFunc(); // protected
st.testRight();
return 0;
}
4. 同名隐藏
class Person{ private: string name; bool gender; int age; public: Person(){ cout << “Person()” << endl; } Person(string name, bool gender, int age){ name = name; gender = gender; age = age; cout << “Person(string, bool, int)” << endl; } void show(){ cout << “本人名叫” << name << endl; cout << (gender == true ? “帅哥一枚” : “美女一枚”) << endl; cout << “年方” << age << “青春无敌”<< endl; } };
class Student : public Person{ private: int number; public: void show(){ Person::show(); // 调用父类的show(); cout << “学号 : “ << number << endl; } };
int main(int argc, const char** argv){ Student st; st.show();
cout << "sizeof(Student) : " << sizeof(Student) << endl;
return 0;
}
<a name="VpKaD"></a>
## 5. 子类构造函数
- 父类部分的初始化,由父类构造函数负责
- 子类新增部分的初始化,由子类构造函数负责
- 默认的子类构造函数:
- 编译器会给子类提供无参构造函数,并自动调用父类的无参构造函数
- 自定义子类构造函数:
- 必须在子类构造函数的初始化列表中指定父类的构造函数
- 构造函数的执行顺序:
- 先父类构造函数,后子类构造函数
- 示例代码:
```cpp
#include <iostream>
using namespace std;
class Person{
private:
string name;
bool gender;
int age;
public:
Person(){
cout << "Person()" << endl;
}
Person(string name_, bool gender_, int age_){
name = name_;
gender = gender_;
age = age_;
cout << "Person(string, bool, int)" << endl;
}
void show(){
cout << "本人名叫" << name << endl;
cout << (gender == true ? "帅哥一枚" : "美女一枚") << endl;
cout << "年方" << age << "青春无敌"<< endl;
}
};
class Student : public Person{
private:
int number;
public:
Student() : Person(){
cout << "Student()" << endl;
}
Student(string name, bool gender, int age, int number) : Person(name, gender, age){
this->number = number;
cout << "Student(string, bool, int, int)" << endl;
}
void show(){
Person::show(); // 调用父类的show();
cout << "学号 : " << number << endl;
}
};
int main(int argc, const char** argv){
Student st;
st.show();
cout << "-------------------" << endl;
Student st1("rose", false, 18, 1907201);
st1.show();
return 0;
}
6. 子类拷贝构造函数
- 默认的子类拷贝构造函数:
- 编译器会提供默认的拷贝构造函数,自动调用父类拷贝构造函数
- 自定义子类拷贝构造函数:
- 必须要在子类拷贝构造函数的初始化列表中指定父类的拷贝构造函数
- 构造函数的执行顺序:
- 先 父类拷贝构造函数, 后 子类拷贝构造函数
- 示例代码:
```cpp
include
using namespace std;
class Person{ private: string name; bool gender; int age; public: Person(){ cout << “Person()” << endl; } Person(string name, bool gender, int age){ name = name; gender = gender; age = age; cout << “Person(string, bool, int)” << endl; } void show(){ cout << “本人名叫” << name << endl; cout << (gender == true ? “帅哥一枚” : “美女一枚”) << endl; cout << “年方” << age << “青春无敌”<< endl; } };
class Student : public Person{ private: int number; public: Student() : Person(){ cout << “Student()” << endl; } Student(string name, bool gender, int age, int number) : Person(name, gender, age){ this->number = number; cout << “Student(string, bool, int, int)” << endl; }
Student(const Student& that) : Person(that){
number = that.number;
cout << "Student(const Student&)" << endl;
}
void show(){
Person::show(); // 调用父类的show();
cout << "学号 : " << number << endl;
}
};
int main(int argc, const char** argv){ Student st; st.show();
cout << "-------------------" << endl;
Student st1("rose", false, 18, 1907201);
st1.show();
cout << "-------------------" << endl;
Student st2(st1);
st2.show();
return 0;
}
<a name="vfg3R"></a>
## 7. 子类析构函数
- 父类部分的清理工作由父类的析构函数负责
- 子类部分的清理工作由子类的析构函数负责
- 父类析构函数由子类析构函数自动调用,无须指定
- 示例代码:
```cpp
#include <iostream>
using namespace std;
class Person{
private:
string name;
bool gender;
int age;
public:
~Person(){
cout << "~Person()" << endl;
}
Person(){
cout << "Person()" << endl;
}
Person(string name_, bool gender_, int age_){
name = name_;
gender = gender_;
age = age_;
cout << "Person(string, bool, int)" << endl;
}
void show(){
cout << "本人名叫" << name << endl;
cout << (gender == true ? "帅哥一枚" : "美女一枚") << endl;
cout << "年方" << age << "青春无敌"<< endl;
}
};
class Student : public Person{
private:
int number;
public:
~Student(){
cout << "~Student()" << endl;
}
Student() : Person(){
cout << "Student()" << endl;
}
Student(string name, bool gender, int age, int number) : Person(name, gender, age){
this->number = number;
cout << "Student(string, bool, int, int)" << endl;
}
void show(){
Person::show(); // 调用父类的show();
cout << "学号 : " << number << endl;
}
};
int main(){
Student st;
st.show();
cout << "-------------------" << endl;
Student st1("rose", false, 18, 1907201);
st1.show();
return 0;
}
8. 子类赋值运算符函数
- 默认的子类赋值运算符函数:
- 编译器会提供默认的赋值运算符函数,该函数会自动调用父类的赋值函数完成父类部分的赋值
- 自定义赋值运算符函数:
- 必须在函数体里显式调用父类的赋值函数
- 示例代码:
```cpp
using namespace std;
include
class Person{ private: string name; bool gender; int age; public: ~Person(){ cout << “~Person()” << endl; } Person(){ cout << “Person()” << endl; } Person(string name, bool gender, int age){ name = name; gender = gender; age = age; cout << “Person(string, bool, int)” << endl; } void show(){ cout << “本人名叫” << name << endl; cout << (gender == true ? “帅哥一枚” : “美女一枚”) << endl; cout << “年方” << age << “青春无敌”<< endl; } };
class Student : public Person{ private: int number; public: ~Student(){ cout << “~Student()” << endl; } Student() : Person(){ cout << “Student()” << endl; } Student(string name, bool gender, int age, int number) : Person(name, gender, age){ this->number = number; cout << “Student(string, bool, int, int)” << endl; }
void show(){
Person::show(); // 调用父类的show();
cout << "学号 : " << number << endl;
}
Student& operator=(const Student& other){
Person::operator=(other);
number = other.number;
cout << "operator=(const Student&)" << endl;
}
};
int main(){ Student st; st.show();
cout << "-------------------" << endl;
Student st1("rose", false, 18, 1907201);
st1.show();
cout << "-------------------" << endl;
st = st1;
st.show();
return 0;
}
<a name="jXuTY"></a>
## 9. 转型
- 向上转型:
- 将子类类型的对象名或者指针的引用转换为父类类型的对象或者指针或引用。这种操作范围缩小的类型转换,在编译器看来是安全的,所以可以隐式转换
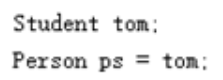
- 向下转型:
- 将父类类型指针或引用转换为子类类型的指针或引用。这种操作范围放大的类型转换,编译器禁止父类对象名强制转换为子类对象名。
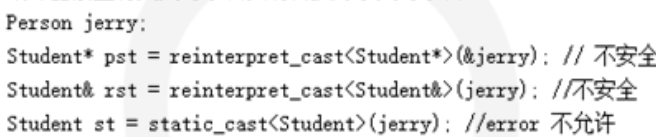
- 示例代码:
```cpp
#include <iostream>
using namespace std;
class Person{
private:
string name;
bool gender;
int age;
public:
Person(){
cout << "Person()" << endl;
}
Person(string name_, bool gender_, int age_){
name = name_;
gender = gender_;
age = age_;
cout << "Person(string, bool, int)" << endl;
}
void show(){
cout << "本人名叫" << name << endl;
cout << (gender == true ? "帅哥一枚" : "美女一枚") << endl;
cout << "年方" << age << "青春无敌"<< endl;
}
};
class Student : public Person{
private:
int number;
public:
Student() : Person(){
cout << "Student()" << endl;
}
Student(string name, bool gender, int age, int number) : Person(name, gender, age){
this->number = number;
cout << "Student(string, bool, int, int)" << endl;
}
void show(){
Person::show(); // 调用父类的show();
cout << "学号 : " << number << endl;
}
};
int main(){
cout << "-------向上转型" << endl;
Student tom("tom", true, 18, 1907201);
Person ps = tom;
Person* pps = &tom;
Person& rps = tom;
cout << "-------向上转型" << endl;
Person jerry("jerry", true, 19);
Student* pst = reinterpret_cast<Student*>(&jerry);
pst->show();
Student& rst = reinterpret_cast<Student&>(jerry);
rst.show();
//Student st = static_cast<Student>(jerry); // error
return 0;
}
10. 多重继承
- 概念:一个子类继承多个父类
- 钻石形继承:
- 多个父类源自同一个”爷类”,称为钻石形继承
- “爷类”在子类中存在多个副本,通过子类对象访问”爷类”成员时会因为存在多个继承路径导致结果是随机的
- 虚继承:
- 为了确保子类中只有一个”爷类”的副本,将父类的继承类型改为虚继承(解决钻石形继承问题的办法)。
class Person{ private: string name; bool gender; int age; public: Person(){ cout << “Person()” << endl; } Person(string name, bool gender, int age){ name = name; gender = gender; age = age; cout << “Person(string, bool, int)” << endl; } void show(){ cout << “本人名叫” << name << endl; cout << (gender == true ? “帅哥一枚” : “美女一枚”) << endl; cout << “年方” << age << “青春无敌”<< endl; } };
class Student : virtual public Person{ public: void study(){ cout << “擅长学习” << endl; } };
class Teacher : virtual public Person{ public: void teaching(){ cout << “擅长教学” << endl; }
};
class Assistant : public Student, public Teacher{ };
int main(){ Assistant ast; ast.teaching(); ast.study();
cout << "sizeof(Person) : " << sizeof(Person) << endl;
cout << "sizeof(Student) : " << sizeof(Student) << endl;
cout << "sizeof(Teacher) : " << sizeof(Teacher) << endl;
cout << "sizeof(Assistant) : " << sizeof(Assistant) << endl;
return 0;
}
<a name="x0U4D"></a>
## 11. 组合
- 在一个类中使用另一个类的对象作为成员,称为**_组合_**
- 实际开发中多继承使用较少,因为它的开发和调试难度都较大,通常使用单继承+组合来替代多继承实现相同功能
- 示例代码:
```cpp
#include <iostream>
using namespace std;
class Person{
private:
string name;
bool gender;
int age;
public:
Person(){
cout << "Person()" << endl;
}
Person(string name_, bool gender_, int age_){
name = name_;
gender = gender_;
age = age_;
cout << "Person(string, bool, int)" << endl;
}
void show(){
cout << "本人名叫" << name << endl;
cout << (gender == true ? "帅哥一枚" : "美女一枚") << endl;
cout << "年方" << age << "青春无敌"<< endl;
}
};
class Student : public Person{
public:
void study(){
cout << "擅长学习" << endl;
}
};
class Teacher : public Person{
public:
void teaching(){
cout << "擅长教学" << endl;
}
};
class Assistant : public Student{
private:
Teacher t;
public:
void teaching(){
t.teaching();
}
};
int main(){
Assistant ast;
ast.teaching();
ast.study();
return 0;
}