绘制火箭,跟随鼠标
//9:24
PImage rocket;
void setup(){
size(600,500);
rocket = loadImage("🚀.png");
pixelDensity(2);
imageMode(CENTER);
}
void draw(){
image(rocket,mouseX,mouseY,68/2,132/2);
}
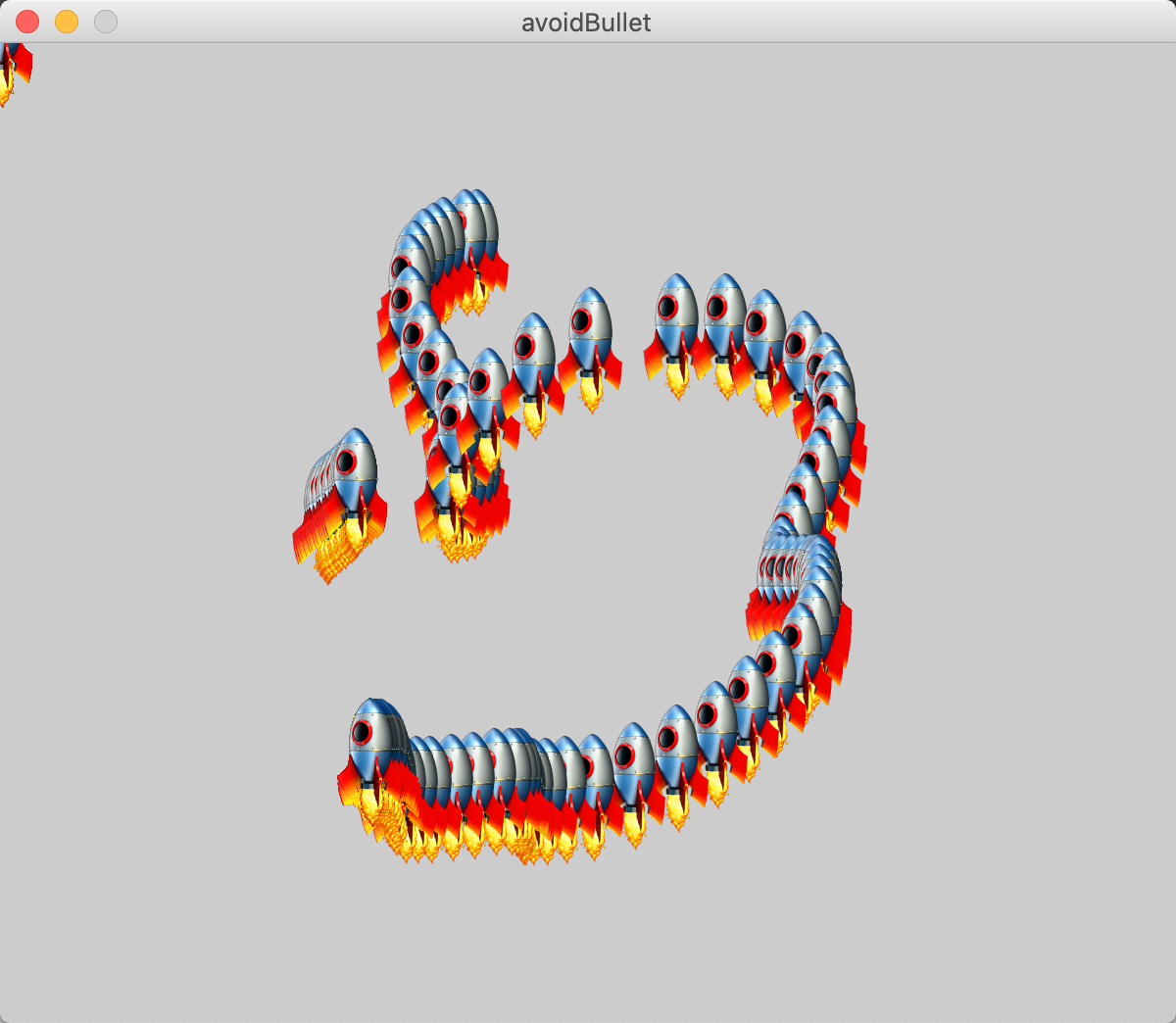
绘制子弹,触壁反弹
//9:24
PImage rocket;
float x, y, r;
float spx, spy;
void setup() {
size(800, 600);
rocket = loadImage("🚀.png");
pixelDensity(2);
imageMode(CENTER);
//variable of bullet
r=10;
x=width/2;
y=r;
spx = 2;
spy=2;
}
void draw() {
background(#132638);
//rocket
image(rocket, mouseX, mouseY, 34, 66);
//bullet
noStroke();
fill(220, 220, 30);
ellipse(x, y, 2*r, 2*r);
//update bullet position
x+=spx;
y+=spy;
//checkEdge
if(x>=width-r || x<r){
spx = -spx;
}else if(y>=height-r || y<r ){
spy = -spy;
}
if(x<mouseX+34/2 && x>mouseX-34 && y>mouseY-66/2 && y<mouseY+66/2){
background(255,100,100);
noLoop();
}
}
模块化
//9:24
PImage rocket;
float x, y, r;
float spx, spy;
void setup() {
size(800, 600);
rocket = loadImage("🚀.png");
pixelDensity(2);
imageMode(CENTER);
//variable of bullet
r=10;
x=width/2;
y=r;
spx = 2;
spy=2;
}
void draw() {
background(#132638);
//rocket
image(rocket, mouseX, mouseY, 34, 66);
display();
update();
checkEdge();
if (x<mouseX+34/2 && x>mouseX-34 && y>mouseY-66/2 && y<mouseY+66/2) {
background(255, 100, 100);
noLoop();
}
}
void display() {
//display bullet
noStroke();
fill(220, 220, 30);
ellipse(x, y, 2*r, 2*r);
}
void update() {
//update bullet position
x+=spx;
y+=spy;
}
void checkEdge() {
//check edge
if (x>=width-r || x<r) {
spx = -spx;
} else if (y>=height-r || y<r ) {
spy = -spy;
}
}
面向对象编程
//9:24
PImage rocket;
Bullet [] bullets= new Bullet[10];
void setup() {
size(800, 600);
rocket = loadImage("🚀.png");
pixelDensity(2);
imageMode(CENTER);
for (int i=0; i<bullets.length; i++) {
bullets[i] = new Bullet();
}
}
void draw() {
background(#132638);
//rocket
image(rocket, mouseX, mouseY, 34, 66);
//操作子弹
for (int i=0; i<bullets.length; i++) {
bullets[i].display();
bullets[i].update();
bullets[i].checkEdge();
bullets[i].checkJoin();
}
}
class Bullet {
//子弹的变量
float x, y, r;
float spx, spy;
Bullet() {
//初始化子弹
r=10;
x=random(0,width);
y=r;
spx = 2;
spy=2;
}
void display() {
//display bullet
noStroke();
fill(220, 220, 30);
ellipse(x, y, 2*r, 2*r);
}
void update() {
//update bullet position
x+=spx;
y+=spy;
}
void checkEdge() {
//check edge
if (x>=width-r || x<r) {
spx = -spx;
} else if (y>=height-r || y<r ) {
spy = -spy;
}
}
void checkJoin() {
if (x<mouseX+34/2 && x>mouseX-34 && y>mouseY-66/2 && y<mouseY+66/2) {
background(255, 100, 100);
noLoop();
}
}
}
优化
...
void draw() {
...
//操作子弹
for (int i=0; i<bullets.length; i++) {
bullets[i].display();
bullets[i].update();
bullets[i].checkEdge();
}
for (int i=0; i<bullets.length; i++) {
bullets[i].checkJoin();
}
}
加入声音
import processing.sound.*;
PImage rocket;
SoundFile explode;
...
void setup() {
...
for (int i=0; i<bullets.length; i++) {
bullets[i] = new Bullet(random(r, width), random(r, height), random(-2, 2), random(-2, 2));
}
explode = new SoundFile(this,"explode.wav");
}
...
class Bullet {
...
void checkJoin() {
if (x<mouseX+34/2 && x>mouseX-34 && y>mouseY-66/2 && y<mouseY+66/2) {
background(255, 100, 100);
explode.play();
noLoop();
}
}
}