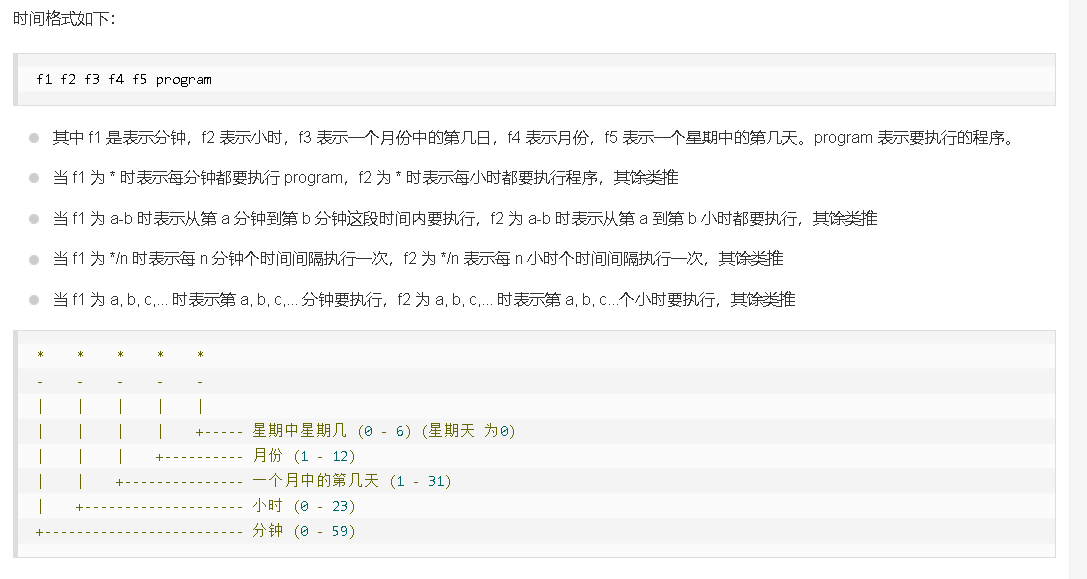
import logging
from crontab import CronTab
from datetime import datetime
import json
from utils.log import log
from utils.jsonfile import JsonFile
class CronOpt:
#创建定时任务
def __init__(self,user="root"):
self.initialize(user)
#初始化
def initialize(self,user="root"):
self.cron = CronTab(user)
#查询列表
def select(self,reInit = False):
if reInit != False:
# 强制重新读取列表
self.initialize()
cronArray = []
for job in self.cron:
# print job.command
schedule = job.schedule(date_from=datetime.now())
cronDict = {
"job" : job,
"task" : (job.command).replace(r'>/dev/null 2>&1', ''),
"next" : str(schedule.get_next()),
"prev" : str(schedule.get_prev()),
"comment": job.comment
}
cronArray.append(cronDict)
return cronArray
#新增定时任务
def add(self, command, timeStr, commentName):
# 创建任务
job = self.cron.new(command=command)
# 设置任务执行周期
job.setall(timeStr)
# 备注,也是命令id
job.set_comment(commentName)
# 写入到定时任务
self.cron.write()
# 返回更新后的列表
return self.select(True)
#删除定时任务
def delCron(self,commentName):
# 创建任务
for job in self.cron :
if job.comment == commentName:
self.cron.remove(job)
self.cron.write()
return self.select(True)
#更新定时任务
def update(self, command, timeStr, commentName):
# 先删除任务
self.delCron(commentName)
# 再创建任务,以达到更新的结果
return self.add(command, timeStr, commentName)
def start(self):
# c = CronOpt()
# print(c.select())
# # log.info(json.loads(c.select()))
# # 新增定时任务
# #print(c.add("ls /home","*/10 * * * *","测试"))
# # 删除定时任务
# # print(c.delCron("测试"))
'''
思路:
读取配置文件
如果系统的定时任务中不存在配置文件中的定时任务id,则新增该定时任务
如果配置文件不存在系统中的定时任务id,则删除该任务
'''
c = CronOpt()
jsonfile = JsonFile()
contabjson = jsonfile.file_to_json("/home/zhanghui/script/lanhui/robot/mycrontab.json")
crontabjsondict = {}
for onecron in contabjson:
crontabjsondict[onecron["commentName"]] = onecron
selectdict = {}
for oneselect in c.select():
selectdict[oneselect["comment"]] = oneselect
if oneselect["comment"] not in crontabjsondict and oneselect["comment"] != "":
# print("删除定时任务")
log.info("删除定时任务:"+str(c.delCron(oneselect["comment"])))
for onecron in contabjson:
if onecron["commentName"] not in selectdict:
# print("增加定时任务")
log.info("增加定时任务:"+str(c.add(onecron["command"],onecron["timeStr"], onecron["commentName"])))