import requests
import re
import json
import os
if __name__ == '__main__':
url = 'https://y.music.163.com/m/album?id=16959'
songURL = '下载地址不公开'
headers = {
'User-Agent': 'Mozilla/5.0 (Linux; Android 6.0; Nexus 5 Build/MRA58N) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.193 Mobile Safari/537.36'
}
response = requests.get(url=url, headers=headers)
if response.status_code == 200:
html = response.content.decode()
# 使用正则表达式提取网易js中的json数据
pattern = re.compile(r'{"Album".*}', re.I | re.M)
js = pattern.findall(html)[0]
json_text = json.loads(js)
songs = json_text['Album']['songs']
songNames = []
if len(songs) > 0:
for song_item in songs:
dict = {}
dict['id'] = songURL.format(song_item['id'])
dict['songName'] = song_item['songName']
songNames.append(dict)
file_path = '许嵩歌曲'
for song in songNames:
url = song['id']
response = requests.get(url=url, headers=headers)
if response.status_code == 200:
data = response.content
try:
if not os.path.exists(file_path):
print('文件夹', file_path, '不存在,重新建立')
os.makedirs(file_path)
# 拼接图片名(包含路径)
filename = '{}/{}'.format(file_path,
song['songName']) + '.mp3'
with open(filename, 'wb')as f:
f.write(data)
print('真正下载歌曲【%s】' % song['songName'])
except IOError as e:
print('文件操作失败:' + e)
except Exception as e:
print('错误:' + e)
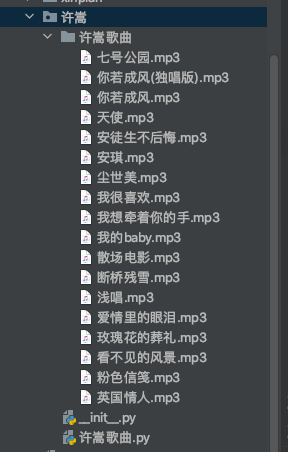