- 快速创建一个表结构相同的表
create table student2 like student;
#查看表结构
desc student;
# 直接删除表
drop table student2 ;
# 判断表是否存在,如果存在则删除表
DROP TABLE IF EXISTS student2; - 添加表列 ADD
alter table student add remark varchar(20); - 修改列类型 MODIFY
alter table student modify remark char(50); - 修改列名 CHANGE
alter table student change remark intro varchar(30); - 删除列 DROP
alter table student drop intro; - 修改表名
rename table student to student2;
# 将 student 表的编码修改成 gbk
alter table student character set utf8; - 创建一张表
create table student(
id int,
name varchar(20),
age int,
sex char(1),
address varchar(30)
) - 插入所有列(七龙珠)
insert into student values (3, ‘孙悟饭’, 18, ‘男’, ‘龟仙人洞中’);
insert into student value (4, ‘克林’, 18, ‘男’, ‘龟仙人洞中’); - 如果只插入部分列,必须写列名(会报错)
insert into student values (3, ‘孙悟饭’, 18, ‘男’);
# 查询student表中的所有数据
select * from student;
# 字符和日期型数据应包含在单引号中
insert into student2 values (10 , ‘小花’ , ‘2020-1-1’);
insert into student2 values (11 , ‘小李’ , now());">插入数据时偷懒的做法
insert into student values (3, ‘孙悟饭饭饭’, null, ‘男’, null);
# 查询student表中的所有数据
select * from student;
# 字符和日期型数据应包含在单引号中
insert into student2 values (10 , ‘小花’ , ‘2020-1-1’);
insert into student2 values (11 , ‘小李’ , now());
# 复制一个一模一样的表结构出来
create table newstudent like student;">查看包含 character 开头的全局变量
show variables like ‘character%’;
# 复制一个一模一样的表结构出来
create table newstudent like student;- 将 student 表中的数据添加到 student2 表中
insert into newstudent select * from student; - 如果只想复制 student 表中 name,age 字段数据到 student2 表中,两张表都写出相应的列名
insert into newstudent (name,age) select name,age from student; - 不带条件修改数据,将所有的性别改成女
update student set sex = ‘男’ , address = ‘黄石市’ ; - 带条件修改数据,将 id 号为 2 的学生性别改成男
update student set address=’深圳’ where id=2;
# 一次修改多个列,把 id 为 3 的学生,年龄改成 26 岁,address 改成北京
update student set age=30, address=’上海’ where id=3; - 不带条件删除数据
delete from student; - 带条件删除数据,删除 id 为 1 的记录
# 可以是数据回滚,也能支持触发器
delete from student where id=1; - 使用 truncate 删除表中所有记录
# truncate 相当于删除表的结构,再创建一张表。它是没有办法进行数据回滚的,也不能支持触发器
TRUNCATE TABLE student;# 基本不用这个
# 查询指定列的数据,多个列之间以逗号分隔
select name , address, sex from student;">查询所有的学生
select * from student ;
# 查询指定列的数据,多个列之间以逗号分隔
select name , address, sex from student;- 表使用别名
selectname
as 姓名 , age as 年龄 from student as st
CREATE TABLE student3 (
id int, — 编号
name varchar(20), — 姓名
age int, — 年龄
sex varchar(5), — 性别
address varchar(100), — 地址
math int, — 数学
english int — 英语
);">清除重复值DISTINCT
# 查询学生来至于哪些地方
select distinct address,age from student;#address和age都相同的行只保留一行
CREATE TABLE student3 (
id int, — 编号
name varchar(20), — 姓名
age int, — 年龄
sex varchar(5), — 性别
address varchar(100), — 地址
math int, — 数学
english int — 英语
);- null不能使用等于 , 只能使用 is 来进行判断
select * from student3 where english is null;
# ——————————————————————————————————— - ————————————————————————————————————
— 查询 age 大于 35 且性别为男的学生(两个条件同时满足)
select * from student3 where age > 35 and sex = ‘男’ and address = ‘香港’; - ————————————————————————————————————
— 查询 id 是 1 或 3 或 5 的学生
select * from student3 where id in(1,3,5); - 查询 english 成绩大于等于 75,且小于等于 90 的学生
select * from student3 where english >= 77 and english <= 90; # 包头又包尾 - LIKE 表示模糊查询
# % 匹配任意多个字符串 0 ~ N
# _ 匹配一个字符 1 ~ 1 - 列出职位为(MANAGER)的员工的编号,姓名
select empno , ename from emp where job = ‘MANAGER’; - 找出奖金高于工资的员工信息
select * from emp where comm > sal ; - 找出每个员工奖金和工资的总和
select * , ifnull(comm,0) + sal as 总工资 from emp ; - 找出部门 10 中的经理(MANAGER)和部门 20 中的普通员工(CLERK)
select * from emp where (deptno = 10 and job = ‘MANAGER’) or (deptno = 20 and job = ‘CLERK’); - 找出部门 10 中既不是经理也不是普通员工,而且工资大于等于 2000 的员工
select * from emp where deptno = 10 and job not in(‘MANAGER’,’CLERK’) and sal > 2000; - 找出有奖金的员工的不同工作
select DISTINCT job from emp where comm is not null; - 找出没有奖金或者奖金低于 500 的员工
select * from emp where comm is null or comm < 500; - 显示雇员姓名,根据其服务年限,将最老的雇员排在最前面
select ename,hiredate from emp order by hiredate asc;
# ——————————————————————————————————————————————————————
快速创建一个表结构相同的表
create table student2 like student;
#查看表结构
desc student;
# 直接删除表
drop table student2 ;
# 判断表是否存在,如果存在则删除表
DROP TABLE IF EXISTS student2;
添加表列 ADD
alter table student add remark varchar(20);
修改列类型 MODIFY
alter table student modify remark char(50);
修改列名 CHANGE
alter table student change remark intro varchar(30);
删除列 DROP
alter table student drop intro;
修改表名
rename table student to student2;
# 将 student 表的编码修改成 gbk
alter table student character set utf8;
创建一张表
create table student(
id int,
name varchar(20),
age int,
sex char(1),
address varchar(30)
)
desc student;
# 插入部分的列,向学生表中
insert into student (id,name
,age,sex) values (1, ‘孙悟空’, 20, ‘男’);
insert into student (id,name
,age,sex) values (2, ‘孙悟天’, 16, ‘男’);
插入所有列(七龙珠)
insert into student values (3, ‘孙悟饭’, 18, ‘男’, ‘龟仙人洞中’);
insert into student value (4, ‘克林’, 18, ‘男’, ‘龟仙人洞中’);
如果只插入部分列,必须写列名(会报错)
insert into student values (3, ‘孙悟饭’, 18, ‘男’);
插入数据时偷懒的做法
insert into student values (3, ‘孙悟饭饭饭’, null, ‘男’, null);
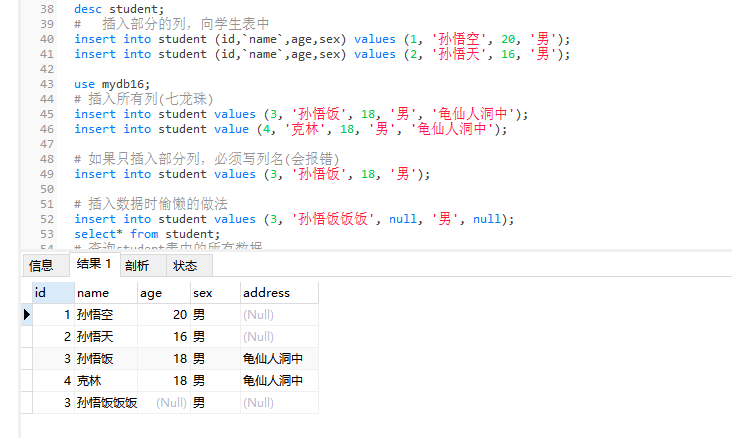
# 查询student表中的所有数据
select * from student;
# 字符和日期型数据应包含在单引号中
insert into student2 values (10 , ‘小花’ , ‘2020-1-1’);
insert into student2 values (11 , ‘小李’ , now());
查看包含 character 开头的全局变量
show variables like ‘character%’;
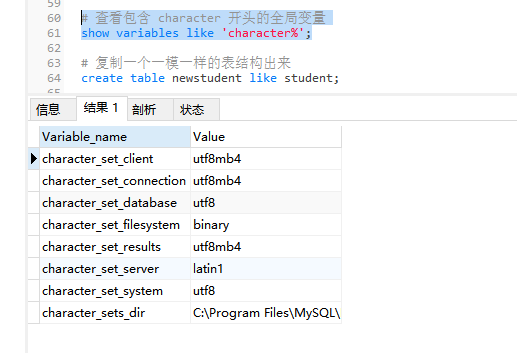
# 复制一个一模一样的表结构出来
create table newstudent like student;
将 student 表中的数据添加到 student2 表中
insert into newstudent select * from student;
如果只想复制 student 表中 name,age 字段数据到 student2 表中,两张表都写出相应的列名
insert into newstudent (name,age) select name,age from student;
不带条件修改数据,将所有的性别改成女
update student set sex = ‘男’ , address = ‘黄石市’ ;
update student set age = age + 1 ;
带条件修改数据,将 id 号为 2 的学生性别改成男
update student set address=’深圳’ where id=2;
# 一次修改多个列,把 id 为 3 的学生,年龄改成 26 岁,address 改成北京
update student set age=30, address=’上海’ where id=3;
update student set age=29, address=’杭州’, sex=’女’ where name = ‘克林’;
不带条件删除数据
delete from student;
带条件删除数据,删除 id 为 1 的记录
# 可以是数据回滚,也能支持触发器
delete from student where id=1;
使用 truncate 删除表中所有记录
# truncate 相当于删除表的结构,再创建一张表。它是没有办法进行数据回滚的,也不能支持触发器
TRUNCATE TABLE student;# 基本不用这个
INSERT INTO student
VALUES (2, ‘孙悟天’, 17, ‘男’, ‘深圳’);
INSERT INTO student
VALUES (3, ‘孙悟饭’, 30, ‘男’, ‘上海’);
INSERT INTO student
VALUES (3, ‘孙悟饭饭’, 30, ‘男’, ‘上海’);
INSERT INTO student
VALUES (3, ‘孙悟饭饭饭’, 30, ‘男’, ‘上海’);
INSERT INTO student
VALUES (4, ‘克林’, 29, ‘女’, ‘杭州’);
查询所有的学生
select * from student ;
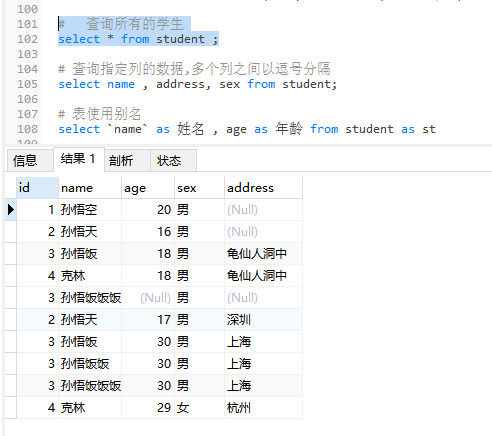
# 查询指定列的数据,多个列之间以逗号分隔
select name , address, sex from student;
表使用别名
select name
as 姓名 , age as 年龄 from student as st
清除重复值DISTINCT
# 查询学生来至于哪些地方
select distinct address,age from student;#address和age都相同的行只保留一行
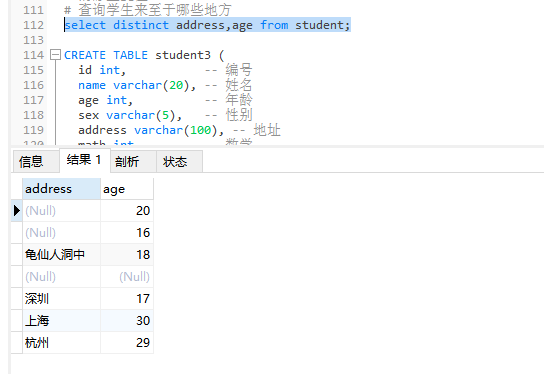
CREATE TABLE student3 (
id int, — 编号
name varchar(20), — 姓名
age int, — 年龄
sex varchar(5), — 性别
address varchar(100), — 地址
math int, — 数学
english int — 英语
);
INSERT INTO student3(id,name
,age,sex,address,math,english) VALUES
(1,’马云’,55,’男’,’杭州’,66,78),
(2,’马化腾’,45,’女’,’深圳’,98,87),
(3,’马景涛’,55,’男’,’香港’,56,77),
(4,’柳岩’,20,’女’,’湖南’,76,65),
(5,’柳青’,20,’男’,’湖南’,86,NULL),
(6,’刘德华’,57,’男’,’香港’,99,99),
(7,’马德’,22,’女’,’香港’,99,99),
(8,’德玛西亚’,18,’男’,’南京’,56,65);
# ———————————————————————————————————
select * from student3;
— 给所有的数学加 5 分
select math , math+5 as 新成绩 from student3;
select ifnull(english,0) from student3;
— 查询 math + english 的和
select *,(math+ ifnull(english,0)) as 总成绩 from student3;— as 可以省略
null不能使用等于 , 只能使用 is 来进行判断
select * from student3 where english is null;
# ———————————————————————————————————
— 查询 math 分数大于 80 分的学生
select * from student3 where math > 80;
— 查询 english 分数小于或等于 80 分的学生
select * from student3 where english <= 78;
— 查询 age 等于 20 岁的学生
select * from student3 where age = 20;
— 查询 age 不等于 20 岁的学生,注:不等于有两种写法
select * from student3 where age <> 20;
select * from student3 where age != 20;
————————————————————————————————————
— 查询 age 大于 35 且性别为男的学生(两个条件同时满足)
select * from student3 where age > 35 and sex = ‘男’ and address = ‘香港’;
— 查询 age 大于 35 或性别为男的学生(两个条件其中一个满足)
select * from student3 where age>35 or sex=’男’;
— 查询 id 是 1 或 3 或 5 的学生
select * from student3 where id=1 or id=3 or id=5;
— 混用 and 优先级 高于 or
select * from student3 where address = ‘香港’ or (age > 35 and sex = ‘男’)
————————————————————————————————————
— 查询 id 是 1 或 3 或 5 的学生
select * from student3 where id in(1,3,5);
— 查询 id 不是 1 或 3 或 5 的学生
select * from student3 where id not in(1,3,5);
select * from student3 where english is not null ;
查询 english 成绩大于等于 75,且小于等于 90 的学生
select * from student3 where english >= 77 and english <= 90; # 包头又包尾
select * from student3 where english between 77 and 90; # 包头又包尾
LIKE 表示模糊查询
# % 匹配任意多个字符串 0 ~ N
# _ 匹配一个字符 1 ~ 1
— 查询姓马的学生
select * from student3 where name like ‘马%’;
select * from student3 where name like ‘马’; # 仅查询名字叫 ‘马’
— 查询姓名中包含’德’字的学生
select * from student3 where name like ‘%德%’;
— 查询姓马,且姓名有两个字的学生
select from student3 where name like ‘马_’;
#\是转义字符,在\后面的%会失去通配符作用,只是一个简单的百分号
select from student3 where name like ‘马\%_\%’;
作业讲解
# 选择在部门 30 中员工的所有信息
select * from emp where deptno = 30 ;