图例
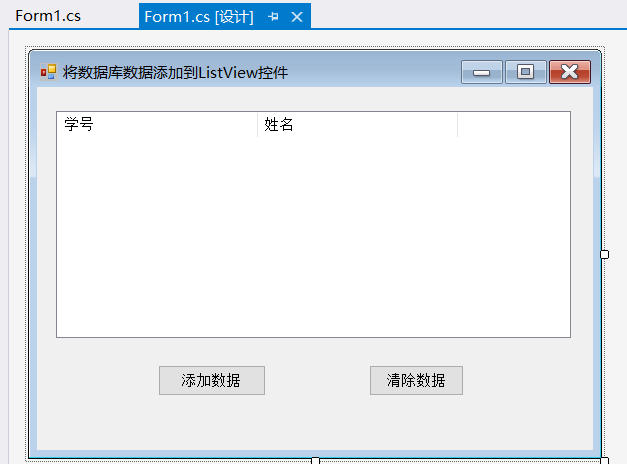
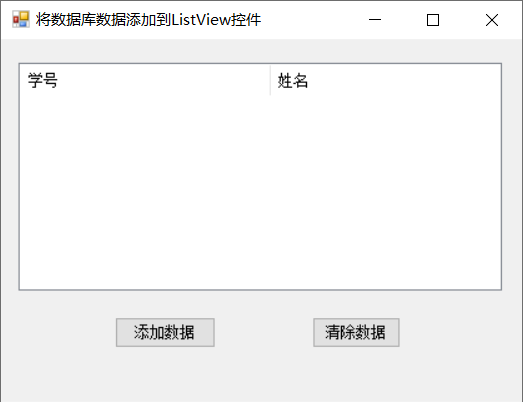
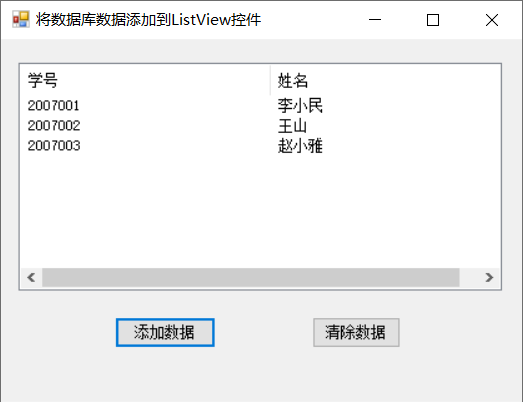
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace DataBind_ListView
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnBindData_Click(object sender, EventArgs e)
{
// 连接数据库
SqlConnection myCon = new SqlConnection();
myCon.ConnectionString = "Data Source=DESKTOP-VFJD28I;" +
"Initial Catalog=db_Class;" +
"Integrated Security=True"; // 这个数据库连接字符串在左侧服务器资源管理器-数据连接下-右击属性找到复制粘贴到这里
myCon.Open();
// 使用 SqlCommand 提交查询命令
SqlCommand selectCMD = new SqlCommand("SELECT top 10 SNo,SName FROM tb_Student", myCon);
// 创建SqlDataReader
SqlDataReader dr = selectCMD.ExecuteReader();
listView1.View = View.Details; // 设置显示模式
listView1.FullRowSelect = true;
// 将 DataReader 中的数据绑定到 ListView1
while (dr.Read())
{
ListViewItem lv = new ListViewItem(dr[0].ToString());
lv.SubItems.Add(dr[1].ToString());
listView1.Items.Add(lv);
}
dr.Close();
// 断开连接
myCon.Close();
}
private void btnClearData_Click(object sender, EventArgs e)
{
listView1.Items.Clear();
}
}
}