简介
概念
设计模式是在特定环境下人们解决某类重复出现问题的一套成功 或有效的解决方案。一开始出现于建筑行业,后来被引入到计算机领域.
软件设计模式,是指在软件设计中,被反复使用的一种代码设计经验。使用设计模式的目的是为了可重用代码,提高代码的可扩展性和可维护性。
设计模式这个术语是上个世纪90年代由Erich Gamma、Richard Helm、Raplh Johnson和Jonhn Vlissides四个人总结提炼出来的,并且写了一本Design Patterns的书。这四人也被称为四人帮(GoF)。
种类
GoF 提出的设计模式有 23 个,包括:
- 创建型(Creational)模式: 如何创建对象;
- 结构型(Structural )模式: 如何实现类或对象的组合;
行为型(Behavioral)模式: 类或对象怎样交互以及怎样分配职责。
有一个“简单工厂模式”不属于 GoF 23 种设计模式,但大部分的设计模式 书籍都会对它进行专门的介绍.
所以,设计模式目前种类: GoF 的 23 种 + “简单工厂模式” = 24 种。
这里只学习一部分比较常用的设计模式,看全部可以—>设计原则
为什么要使用设计模式?根本原因还是软件开发要实现可维护、可扩展,就必须尽量复用代码,并且降低代码的耦合度。(高内聚,低耦合)设计模式主要是基于OOP编程提炼的,它基于以下几个原则:
单一职责原则: 类的职责单一,对外只提供一种功能,而引起类变化的 原因都应该只有一个
- 开闭原则:软件应该对扩展开放,而对修改关闭。这里的意思是在增加新功能的时候,能不改代码就尽量不要改,如果只增加代码就完成了新功能,那是最好的。
- 里氏替换原则: 任何抽象类出现的地方都可以用他的实现类进行替换, 实际就是虚拟机制,语言级别实现面向对象功能.
- 接口隔离原则: 不应该强迫用户的程序依赖他们不需要的接口方法。一 个接口应该只提供一种对外功能,不应该把所有操作都 封装到一个接口中去
- 合成复用原则: 如果使用继承,会导致父类的任何变换都可能影响到子 类的行为。如果使用对象组合,就降低了这种依赖关系。 对于继承和组合,优先使用组合
- 迪米特法则:一个对象应当对其他对象尽可能少的了解,从而降 低各个对象之间的耦合,提高系统的可维护性。 ```cpp //开闭原则:新增功能,尽量不修改代码,而是增加代码 class Caculator { private: double mA; string mOpt; double mB;
public: Caculator(double a, string opt, double b) : mA(a), mOpt(opt), mB(b) {} double getResult() { if (this->mOpt == “+”) { return this->mA + this->mB; } else if (this->mOpt == “-“) { return this->mA - this->mB; } //… } }; //开闭原则写法—> class AbstractCaculator { public: double mA; double mB;
public: AbstractCaculator(double a, double b) : mA(a), mB(b) {} virtual double getResult() = 0; };
class PlusCaculator : public AbstractCaculator { public: PlusCaculator(double a, double b) : AbstractCaculator(a,b){} double getResult() { return mA + mB; } }; class MinusCaculator : public AbstractCaculator { public: MinusCaculator(double a, double b) : AbstractCaculator(a,b){} double getResult() { return mA - mB; } }; //…使用这种写法,可扩展性强
其他案例:<br />迪米特原则(最少知识原则):租房客有很多出租屋可选择.租房客只需要租金..信息,不需要知道建筑材料..信息,可添加一个中介,提供给租房客需要的信息和服务.<br />合成复用原则:优先使用组合而不是继承<br />依赖倒转原则:传统高层依赖于底层,耦合性高,在中间加入抽象层.
<a name="RGZVQ"></a>
## 创建型模型
<a name="og80m"></a>
### 1.简单工厂模式
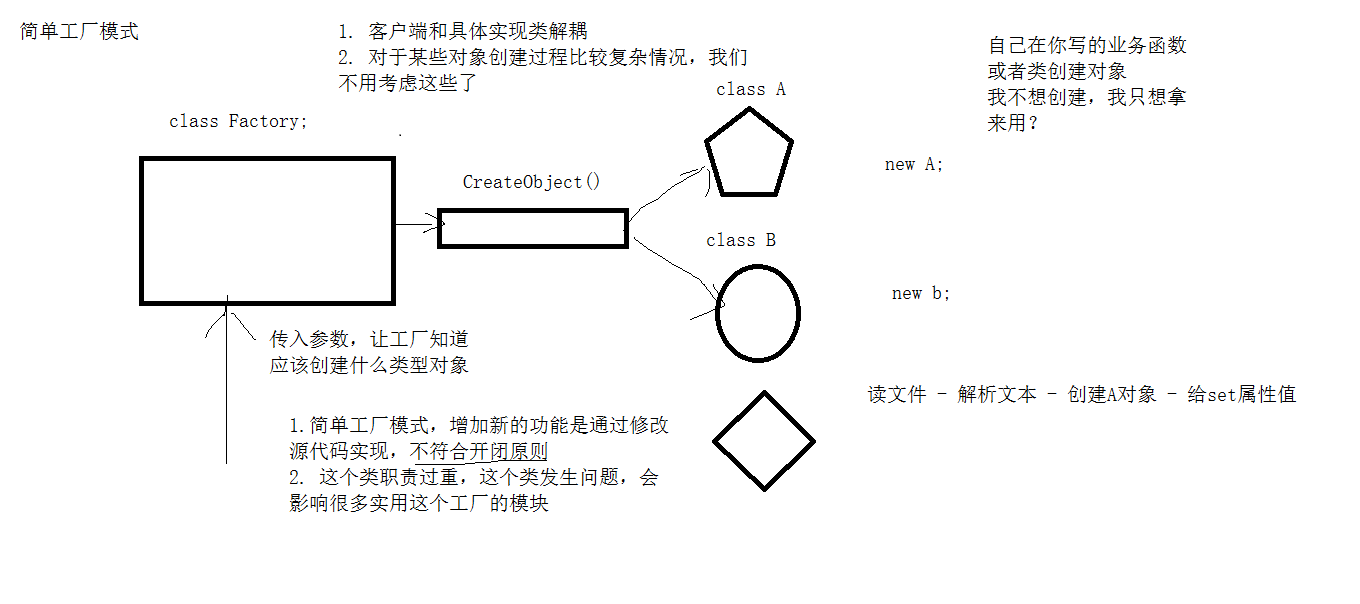
```cpp
#include <iostream>
using namespace std;
class AbstractPhone
{
public:
virtual void show() = 0;
};
class HuaWei : public AbstractPhone
{
public:
void show()
{
cout << "我是爱国牌手机!" << endl;
}
};
class RedMi : public AbstractPhone
{
public:
void show()
{
cout << "我是红米,干翻小米!" << endl;
}
};
class PhoneFactory
{
public:
static AbstractPhone *CreateObject(string name)
{
if (name == "huawei")
{
return new HuaWei;
}
else if (name == "redmi")
{
return new RedMi;
}
}
};
int main(int argc, char const *argv[])
{
AbstractPhone * phone = NULL;
phone = PhoneFactory::CreateObject("huawei");
phone->show();
delete phone;
phone = NULL;
return 0;
}
2. 工厂方法模式
#include <iostream>
using namespace std;
class AbstractPhone
{
public:
virtual void show() = 0;
};
class HuaWei : public AbstractPhone
{
public:
void show()
{
cout << "我是爱国牌手机!" << endl;
}
};
class RedMi : public AbstractPhone
{
public:
void show()
{
cout << "我是红米,干翻小米!" << endl;
}
};
class AbstractFactory
{
public:
virtual AbstractPhone *CreateObject() = 0;
};
class HuaweiFactory : public AbstractFactory
{
public:
AbstractPhone *CreateObject()
{
return new HuaWei;
}
};
class RedMiFactory : public AbstractFactory
{
public:
AbstractPhone *CreateObject()
{
return new RedMi;
}
};
int main(int argc, char const *argv[])
{
AbstractPhone *phone = NULL;
AbstractFactory *factroy = new HuaweiFactory;
phone = factroy->CreateObject();
phone->show();
delete phone;
phone = NULL;
delete factroy;
factroy = NULL;
return 0;
}
3.抽象工厂模式
由于工厂方法模式中的每个工厂只生产一类产品,可能会导致系统 中存在大量的工厂类,势必会增加系统的开销。此时,我们可以考虑将一些相关 的产品组成一个“产品族,由同一个工厂来统一生产 .
//在以上案例扩展,假如每个品牌的工厂都生产两类手机,高端和低端.
//华为高端机,华为低端机,红米高端机,红米低端机.
//再定义一种抽象工厂类,包括生产高端,生产低端的方法
#include <iostream>
using namespace std;
class AbstractPhone
{
public:
virtual void show() = 0;
};
class HuaWeiHigh : public AbstractPhone
{
public:
virtual void show()
{
cout << "我是爱国牌高端手机!" << endl;
}
};
class HuaWeiLow : public AbstractPhone
{
public:
virtual void show()
{
cout << "我是爱国牌低端手机!" << endl;
}
};
class RedMiHigh : public AbstractPhone
{
public:
virtual void show()
{
cout << "我是红米高端机" << endl;
}
};
class RedMiLow : public AbstractPhone
{
public:
virtual void show()
{
cout << "我是红米低端机!" << endl;
}
};
class AbstractFactory
{
public:
virtual AbstractPhone *CreateHigh() = 0;
virtual AbstractPhone *CreateLow() = 0;
};
class HuaweiFactory : public AbstractFactory
{
public:
AbstractPhone *CreateHigh()
{
return new HuaWeiHigh;
}
AbstractPhone *CreateLow()
{
return new HuaWeiLow;
}
};
class RedMiFactory : public AbstractFactory
{
public:
AbstractPhone *CreateHigh()
{
return new RedMiHigh;
}
AbstractPhone *CreateLow()
{
return new RedMiLow;
}
};
int main(int argc, char const *argv[])
{
AbstractPhone *phone = NULL;
AbstractFactory *factroy = new HuaweiFactory;
phone = factroy->CreateHigh();
phone->show();
delete phone;
phone = NULL;
delete factroy;
factroy = NULL;
return 0;
}
4.生成器模式
将一个复杂的对象的构建(builder)和它的表示(director)分离,使得同样的构建过程可以创建不同的表示。核心是给指导者一个生成器,但具体方式不指定。
#include <iostream>
#include <list>
using namespace std;
//产品类,由多个部件组成
class Product
{
private:
list<string> parts;
public:
// 添加产品部件
void add(string part)
{
parts.push_back(part);
}
// 列举所有的产品部件
void show()
{
cout << "---产品 创建---"<<parts.size() << endl;
for (string part : parts)
{
cout << part << endl;
}
}
};
//抽象类或接口
class Builder
{
public:
Product product;
Product getProduct()
{
return product;
}
virtual void buildPartA() = 0;
virtual void buildPartB() = 0;
};
//指挥者类,用来指挥建造过程
class Director
{
public:
void construct(Builder * builder)
{
builder->buildPartA();
builder->buildPartB();
}
};
//具体建造者类
class ConcreteBuilder1 : public Builder
{
public:
virtual void buildPartA()
{
product.add("部件A");
}
virtual void buildPartB()
{
product.add("部件B");
}
};
// 具体建造者类,建造的对象时Product,通过build使Product完善
class ConcreteBuilder2 : public Builder
{
public:
void buildPartA()
{
product.add("部件X");
}
void buildPartB()
{
product.add("部件Y");
}
};
//建造客户端
int main(int argc, char const *argv[])
{
Director director;
Builder * builder1 = new ConcreteBuilder1;
Builder * builder2 = new ConcreteBuilder2;
director.construct(builder1);
Product product1 = builder1->getProduct();
product1.show();
director.construct(builder2);
Product product2 = builder2->getProduct();
product2.show();
return 0;
}
5.单例模式
通过单例模式可以保证系统中一个类只有一个实例而且该实例易 于外界访问,从而方便对实例个数的控制并节约系统资源。如果希望在系统中某 个类的对象只能存在一个,单例模式是最好的解决方案。
实现步骤:
- 构造方法私有,不给new
- 提供静态方法返回唯一的对象
```cpp
//懒汉式:线程不安全
include
using namespace std; class singleton { private: singleton(){}//不给new static singleton s_singleton; public: static singleton getInstance(){
}if(s_singleton == NULL){//多线程时,可能同时判断为NULL
s_singleton = new singleton;
}
return s_singleton;
}; singleton singleton::s_singleton = NULL; int main(int argc, char const argv[]) { singleton s1 = singleton::getInstance(); singleton s2 = singleton::getInstance();
cout<<"同一对象?"<<(s1==s2?"是":"否")<<endl;
return 0;
} //饿汉式
include
using namespace std; class singleton { private: singleton(){}//不给new static singleton s_singleton; public: static singleton getInstance(){ return s_singleton; }
}; singleton singleton::s_singleton = new singleton;//区别处 int main(int argc, char const argv[]) { singleton s1 = singleton::getInstance(); singleton s2 = singleton::getInstance();
cout<<"同一对象?"<<(s1==s2?"是":"否")<<endl;
return 0;
}
<a name="Ji5Ex"></a>
## 结构型模式
<a name="EkZey"></a>
### 6.代理模式
代理模式的定义:为其他对象提供一种代理以控制对这个对象的访问。在某 些情况下,一个对象不适合或者不能直接引用另一个对象,而代理对象可以在客 户端和目标对象之间起到中介的作用。
```cpp
#include <iostream>
using namespace std;
class AbstractCommonInterface
{
public:
virtual void run() = 0;
};
class System : public AbstractCommonInterface
{
public:
void run()
{
cout << "系统功能" << endl;
}
};
class systemProxy : public AbstractCommonInterface
{
public:
System *system;
bool isOK()
{
return true; //验证权限,这里直接给通过
}
void run()
{
if (isOK())
{
this->system = new System;
this->system->run();
}
}
~systemProxy()
{
if (this->system != NULL)
{
delete this->system;
}
}
};
int main(int argc, char const *argv[])
{
systemProxy sProxy;
sProxy.run();
return 0;
}
7.外观模式
Facade 模式也叫外观模式,是由 GoF 提出的 23 种设计模式中的一种。 Facade 模式为一组具有类似功能的类群,比如类库,子系统等等,提供一个一 致的简单的界面。这个一致的简单的界面被称作 facade
#include <iostream>
using namespace std;
//电视
class Television
{
public:
void On()
{
cout << "电视打开!" << endl;
}
void Off()
{
cout << "电视关闭!" << endl;
}
};
//灯
class Lamp
{
public:
void On()
{
cout << "灯打开!" << endl;
}
void Off()
{
cout << "灯关闭!" << endl;
}
};
//音响
class Audio
{
public:
void On()
{
cout << "音响打开!" << endl;
}
void Off()
{
cout << "音响关闭!" << endl;
}
};
//麦克风
class Microphone
{
public:
void On()
{
cout << "麦克风打开!" << endl;
}
void Off()
{
cout << "麦克风关闭!" << endl;
}
};
// DVD 播放机
class DVDPlayer
{
public:
void On()
{
cout << "DVDPlayer 打开!" << endl;
}
void Off()
{
cout << "DVDPlayer 关闭!" << endl;
}
};
//游戏机
class GameMachine
{
public:
void On()
{
cout << "游戏机打开!" << endl;
}
void Off()
{
cout << "游戏界关闭!" << endl;
}
};
//外观抽象类
class AbstractFacede
{
public:
virtual void On() = 0;
virtual void Off() = 0;
};
//游戏模式
class GameMode : public AbstractFacede
{
public:
GameMode()
{
pTelevision = new Television;
pAudio = new Audio;
pGameMachine = new GameMachine;
}
virtual void On()
{
pTelevision->On();
pAudio->On();
pGameMachine->On();
}
virtual void Off()
{
pTelevision->Off();
pAudio->Off();
pGameMachine->Off();
}
private:
Television *pTelevision;
Audio *pAudio;
GameMachine *pGameMachine;
};
// KTV 模式
class KTVMode : public AbstractFacede
{
public:
KTVMode()
{
pTelevision = new Television;
pAudio = new Audio;
pDVDPlayer = new DVDPlayer;
pLamp = new Lamp;
pMicrophone = new Microphone;
}
virtual void On()
{
pTelevision->On();
pAudio->On();
pDVDPlayer->On();
pLamp->Off();
pMicrophone->On();
}
virtual void Off()
{
pTelevision->Off();
pAudio->Off();
pDVDPlayer->Off();
pLamp->On();
pMicrophone->Off();
}
private:
Television *pTelevision;
Audio *pAudio;
DVDPlayer *pDVDPlayer;
Lamp *pLamp;
Microphone *pMicrophone;
};
//测试游戏模式和 KTV 模式
void test01()
{
AbstractFacede *facede = NULL;
facede = new GameMode;
facede->On(); //启动游戏模式
delete facede;
cout << "--------------------------" << endl;
facede = new KTVMode;
facede->On(); //启动 KTV 模式
delete facede;
}
int main()
{
test01();
return EXIT_SUCCESS;
}
8.适配器模式
将一个类的接口转换成客户希望的另外一个接口。使得原本由于接口不兼容 而不能一起工作的那些类可以一起工作。
#include <iostream>
#include <list>
using namespace std;
// A 需要治疗感冒
class PersonA
{
public:
void treatGanmao()
{
cout << "A 需要治疗感冒!" << endl;
}
};
// B 需要治疗头疼
class PersonB
{
public:
void treatTouteng()
{
cout << "B 需要治疗头疼!" << endl;
}
};
//目标接口
class Target
{
public:
virtual void treat() = 0;
};
//将 PersonA 的 treatGanmao 接口适配成 treat
class AdapterPersonA : public Target
{
public:
AdapterPersonA()
{
pPerson = new PersonA;
}
virtual void treat()
{
pPerson->treatGanmao();
}
private:
PersonA *pPerson;
};
//将 PersonB 的 treatTouteng 接口适配成 treat
class AdapterPersonB : public Target
{
public:
AdapterPersonB()
{
pPerson = new PersonB;
}
virtual void treat()
{
pPerson->treatTouteng();
}
private:
PersonB *pPerson;
};
//医生
class Doctor
{
public:
void addPatient(Target *patient)
{
m_list.push_back(patient);
}
void startTreat()
{
for (list<Target *>::iterator it = m_list.begin(); it != m_list.end(); it++)
{
(*it)->treat();
}
}
private:
list<Target *> m_list;
};
void test01()
{
//创建三个病人
Target *patient1 = new AdapterPersonA;
Target *patient2 = new AdapterPersonB;
//创建医生
Doctor *doctor = new Doctor;
doctor->addPatient(patient1);
doctor->addPatient(patient2);
//医生逐个对病人进行治疗
doctor->startTreat();
}
int main()
{
test01();
return EXIT_SUCCESS;
}
9.装饰器模式
装饰模式又叫包装模式,通过一种对客户端透明的方式来扩展对象功能,是 继承关系的一种替代。 装饰模式就是把要附加的功能分别放在单独的类中,并让这个类包含它要装 饰的对象,当需要执行时,客户端就可以有选择的、按顺序的使用装饰功能包装 对象。
#include <iostream>
#include <string>
using namespace std;
//抽象手机类
class AbstractCellphone
{
public:
virtual void showPhone() = 0;
};
//小米手机
class XiaomiCellphone : public AbstractCellphone
{
public:
XiaomiCellphone(string model)
{
m_name = "小米 " + model + "手机";
}
virtual void showPhone()
{
cout << m_name << endl;
}
private:
string m_name;
};
// Apple 手机
class AppleCellphone : public AbstractCellphone
{
public:
AppleCellphone(string model)
{
m_name = "苹果 " + model + "手机";
}
virtual void showPhone()
{
cout << m_name << endl;
}
private:
string m_name;
};
//装饰类
class Decorator : public AbstractCellphone
{
public:
Decorator(AbstractCellphone *cellphone)
{
pCellphone = cellphone;
}
virtual void showPhone()
{
pCellphone->showPhone();
}
protected:
AbstractCellphone *pCellphone;
};
//给手机贴膜
class CellphoneFilm : public Decorator
{
public:
CellphoneFilm(AbstractCellphone *cellphone) : Decorator(cellphone) {}
void addCellphoneFilm()
{
cout << "手机已贴膜!" << endl;
}
virtual void showPhone()
{
addCellphoneFilm();
pCellphone->showPhone();
}
};
//给手机装上手机壳
class CellphoneShell : public Decorator
{
public:
CellphoneShell(AbstractCellphone *cellphone) : Decorator(cellphone) {}
void addShell()
{
cout << "手机已装上保护壳!" << endl;
}
virtual void showPhone()
{
addShell();
pCellphone->showPhone();
}
};
void test01()
{
//创建小米手机
AbstractCellphone *cellphone = NULL;
cellphone = new XiaomiCellphone("Note 女神版");
cellphone->showPhone();
cout << "-----------------------------" << endl;
//给小米手机贴膜
Decorator *cellphoneFilm = new CellphoneFilm(cellphone);
cellphoneFilm->showPhone();
cout << "-----------------------------" << endl;
//给贴膜的小米手机再装上保护壳
Decorator *cellphoneShell = new CellphoneShell(cellphoneFilm);
cellphoneShell->showPhone();
delete cellphoneShell;
delete cellphoneFilm;
delete cellphone;
}
int main()
{
test01();
system("pause");
return EXIT_SUCCESS;
}
行为型模式
10.模板方法模式
定义一个操作中算法的框架,而将一些步骤延迟到子类中。 也就是基类定义了有固定步骤的抽象方法,各派生类实现不同功能
#include<iostream>
using namespace std;
class DrinkTemplate{
public:
//煮水
virtual void BoildWater() = 0;
//冲泡
virtual void Brew() = 0;
//倒入杯中
virtual void PourInCup() = 0;
//加辅助料
virtual void AddSomething() = 0;
//模板方法
void Make(){
BoildWater();
Brew();
PourInCup();
AddSomething();
}
};
//冲泡咖啡
class Coffee : public DrinkTemplate{
public:
virtual void BoildWater(){
cout << "煮山泉水..." << endl;
}
//冲泡
virtual void Brew(){
cout << "冲泡咖啡..." << endl;
}
//倒入杯中
virtual void PourInCup(){
cout << "咖啡倒入杯中..." << endl;
}
//加辅助料
virtual void AddSomething(){
cout << "加糖,加牛奶,加点醋..." << endl;
}
};
//冲泡茶水
class Tea : public DrinkTemplate{
public:
virtual void BoildWater(){
cout << "煮自来水..." << endl;
}
//冲泡
virtual void Brew(){
cout << "冲泡铁观音..." << endl;
}
//倒入杯中
virtual void PourInCup(){
cout << "茶水倒入杯中..." << endl;
}
//加辅助料
virtual void AddSomething(){
cout << "加糖..加柠檬...加生姜..." << endl;
}
};
void test01(){
Tea* tea = new Tea;
tea->Make();
cout << "-----------" << endl;
Coffee* coffee = new Coffee;
coffee->Make();
}
int main(){
test01();
system("pause");
return 0;
}
11.策略模式
#include <iostream>
using namespace std;
//抽象武器 武器策略
class WeaponStrategy{
public:
virtual void UseWeapon() = 0;
};
class Knife : public WeaponStrategy{
public:
virtual void UseWeapon(){
cout << "使用匕首!" << endl;
}
};
class AK47 :public WeaponStrategy{
public:
virtual void UseWeapon(){
cout << "使用AK47!" << endl;
}
};
class Character {
public:
void setWeapon(WeaponStrategy* weapon){
this->pWeapon = weapon;
}
void ThrowWeapon(){
this->pWeapon->UseWeapon();
}
public:
WeaponStrategy* pWeapon;
};
void test01(){
//创建角色
Character* character = new Character;
//武器策略
WeaponStrategy* knife = new Knife;
WeaponStrategy* ak47 = new AK47;
character->setWeapon(knife);
character->ThrowWeapon();
character->setWeapon(ak47);
character->ThrowWeapon();
delete ak47;
delete knife;
delete character;
}
int main(void){
test01();
return 0;
}
12.命令模式
#include <iostream>
#include<queue>
//#include<Windows.h>
using namespace std;
//协议处理类
class HandleClientProtocol{
public:
//处理增加金币
void AddMoney(){
cout << "给玩家增加金币!" << endl;
}
//处理增加钻石
void AddDiamond(){
cout << "给玩家增加钻石!" << endl;
}
//处理玩家装备
void AddEquipment(){
cout << "给玩家穿装备!" << endl;
}
//处理玩家升级
void addLevel(){
cout << "给玩家升级!" << endl;
}
};
//命令接口
class AbstractCommand{
public:
virtual void handle() = 0; //处理客户端请求的接口
};
//处理增加金币请求
class AddMoneyCommand :public AbstractCommand{
public:
AddMoneyCommand(HandleClientProtocol* protocol){
this->pProtocol = protocol;
}
virtual void handle(){
this->pProtocol->AddMoney();
}
public:
HandleClientProtocol* pProtocol;
};
//处理增加钻石的请求
class AddDiamondCommand :public AbstractCommand{
public:
AddDiamondCommand(HandleClientProtocol* protocol){
this->pProtocol = protocol;
}
virtual void handle(){
this->pProtocol->AddDiamond();
}
public:
HandleClientProtocol* pProtocol;
};
//处理玩家穿装备的请求
class AddEquipmentCommand : public AbstractCommand{
public:
AddEquipmentCommand(HandleClientProtocol* protocol){
this->pProtocol = protocol;
}
virtual void handle(){
this->pProtocol->AddEquipment();
}
public:
HandleClientProtocol* pProtocol;
};
//处理玩家升级的请求
class AddLevelCommand : public AbstractCommand{
public:
AddLevelCommand(HandleClientProtocol* protocol){
this->pProtocol = protocol;
}
virtual void handle(){
this->pProtocol->addLevel();
}
public:
HandleClientProtocol* pProtocol;
};
//服务器程序
class Serser{
public:
void addRequest(AbstractCommand* command){
mCommands.push(command);
}
void startHandle(){
while (!mCommands.empty()){
//Sleep(2000);
AbstractCommand* command = mCommands.front();
command->handle();
mCommands.pop();
}
}
public:
queue<AbstractCommand*> mCommands;
};
void test01(){
HandleClientProtocol* protocol = new HandleClientProtocol;
//客户端增加金币的请求
AbstractCommand* addmoney = new AddMoneyCommand(protocol);
//客户端增加钻石的请求
AbstractCommand* adddiamond = new AddDiamondCommand(protocol);
//客户端穿装备的请求
AbstractCommand* addequpment = new AddEquipmentCommand(protocol);
//客户端升级请求
AbstractCommand* addlevel = new AddLevelCommand(protocol);
Serser* server = new Serser;
//将客户端请求加入到处理的队列中
server->addRequest(addmoney);
server->addRequest(adddiamond);
server->addRequest(addequpment);
server->addRequest(addlevel);
//服务器开始处理请求
server->startHandle();
}
int main(void){
test01();
return 0;
}
13.观察者模式
观察者模式是用于建立一种对象与对象之间的依赖关系,一个对象发生改变 时将自动通知其他对象,其他对象将相应作出反应。在观察者模式中,发生改变 的对象称为观察目标,而被通知的对象称为观察者,一个观察目标可以对应多个 观察者,而且这些观察者之间可以没有任何相互联系,可以根据需要增加和删除 观察者,使得系统更易于扩展。
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include<list>
using namespace std;
//抽象的英雄 抽象的观察者
class AbstractHero{
public:
virtual void Update() = 0;
};
//具体英雄 具体观察者
class HeroA :public AbstractHero{
public:
HeroA(){
cout << "英雄A正在撸BOSS ..." << endl;
}
virtual void Update(){
cout << "英雄A停止撸,待机状态..." << endl;
}
};
class HeroB :public AbstractHero{
public:
HeroB(){
cout << "英雄B正在撸BOSS ..." << endl;
}
virtual void Update(){
cout << "英雄B停止撸,待机状态..." << endl;
}
};
class HeroC :public AbstractHero{
public:
HeroC(){
cout << "英雄C正在撸BOSS ..." << endl;
}
virtual void Update(){
cout << "英雄C停止撸,待机状态..." << endl;
}
};
class HeroD :public AbstractHero{
public:
HeroD(){
cout << "英雄D正在撸BOSS ..." << endl;
}
virtual void Update(){
cout << "英雄D停止撸,待机状态..." << endl;
}
};
class HeroE :public AbstractHero{
public:
HeroE(){
cout << "英雄E正在撸BOSS ..." << endl;
}
virtual void Update(){
cout << "英雄E停止撸,待机状态..." << endl;
}
};
//观察目标抽象
class AbstractBoss{
public:
//添加观察者
virtual void addHero(AbstractHero* hero) = 0;
//删除观察者
virtual void deleteHero(AbstractHero* hero) = 0;
//通知所有观察者
virtual void notify() = 0;
};
//具体的观察者 BOSSA
class BOSSA : public AbstractBoss{
public:
virtual void addHero(AbstractHero* hero){
pHeroList.push_back(hero);
}
//删除观察者
virtual void deleteHero(AbstractHero* hero){
pHeroList.remove(hero);
}
//通知所有观察者
virtual void notify(){
for (list<AbstractHero*>::iterator it = pHeroList.begin(); it != pHeroList.end();it ++){
(*it)->Update();
}
}
public:
list<AbstractHero*> pHeroList;
};
void test01(){
//创建观察者
AbstractHero* heroA = new HeroA;
AbstractHero* heroB = new HeroB;
AbstractHero* heroC = new HeroC;
AbstractHero* heroD = new HeroD;
AbstractHero* heroE = new HeroE;
//创建观察目标
AbstractBoss* bossA = new BOSSA;
bossA->addHero(heroA);
bossA->addHero(heroB);
bossA->addHero(heroC);
bossA->addHero(heroD);
bossA->addHero(heroE);
cout << "heroC阵亡..." << endl;
bossA->deleteHero(heroC);
cout << "Boss死了...通知其他英雄停止攻击,抢装备..." << endl;
bossA->notify();
}
int main(void){
test01();
return 0;
}