1. Cordra服务端下载使用(windows系统下)
- 下载地址:cordra-2.3.0
- 部署Cordra时有很多选项可配置。最简单的是一个独立的Cordra服务,它使用本地文件系统存储bdbje和一个嵌入式索引器lucene。同时也是默认的模式配置,默认模式方便用来测试Cordra功能或使用Cordra构建任何应用程序。
解压下载的zip文件,进入cordra-2.3.0\data路径下,修改repoInit.json初始配置文件,设置初始的密码和标识句柄前缀,从该前缀将生成唯一标识符;如果不设置前缀,则默认情况下将使用测试用前缀来创建对象。
{
"adminPassword": "admin123",
"prefix": "86.1234.abc.TEST"
}
回退到cordra-2.3.0路径下,点击startup.bat启动服务,可以看到服务的访问路径http://127.0.0.1:8080/和https协议的访问路径https://127.0.0.1:8443
写一个java测试项目,springboot框架工具下导入pom依赖。因cordra包内的httpclient需要较高版本,最好带上对应的httpclient依赖,避免和spring-boot-starter-web依赖起冲突;gson依赖同理
<dependency>
<groupId>net.cnri.cordra</groupId>
<artifactId>cordra-client</artifactId>
<version>2.2.0</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.12</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
application.properties配置启动端口号,因为8080被cordra服务占用,改用其它端口号,如8081;另外因为使用了gson,所以需要配置对应的spring转换配置
server.port=8081
spring.http.converters.preferred-json-mapper=gson
#Spring Boot >= 2.3.0.RELEASE情况下用下面配置
#spring.mvc.converters.preferred-json-mapper=gson
写一段Restful风格测试代码,CRUD数字对象 ```java package com.dtdream.test.cordra;
import net.cnri.cordra.api.CordraClient; import net.cnri.cordra.api.CordraException; import net.cnri.cordra.api.CordraObject; import net.cnri.cordra.api.SearchResults; import net.cnri.cordra.api.TokenUsingHttpCordraClient; import org.springframework.web.bind.annotation.DeleteMapping; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController;
import java.util.List; import java.util.stream.Collectors;
@RestController public class CordraController {
static CordraClient cordra;
static {
try {
cordra = new TokenUsingHttpCordraClient("https://127.0.0.1:8443", "admin", "admin123");
} catch (CordraException e) {
e.printStackTrace();
}
}
/**
* 新增
*
* @param
* @return
*/
@PostMapping
public CordraObject create(@RequestBody CordraObject cordraObject) throws CordraException {
return cordra.create(cordraObject);
}
/**
* 获取单个对象
*
* @param
* @return
*/
@GetMapping
public CordraObject get(@RequestParam String id) throws CordraException {
return cordra.get(id);
}
/**
* 获取所有对象
*
* @param
* @return
*/
@GetMapping("all")
public List<CordraObject> getAll() throws CordraException {
SearchResults<CordraObject> results = cordra.search("*:*");
return results.stream().collect(Collectors.toList());
}
/**
* 更新单个对象
*
* @param
* @return
*/
@PutMapping
public CordraObject update(@RequestBody CordraObject cordraObject) throws CordraException {
return cordra.update(cordraObject);
}
/**
* 删除单个对象
*
* @param
* @return
*/
@DeleteMapping
public CordraObject delete(@RequestParam String id) throws CordraException {
cordra.delete(id);
return cordra.get(id);
}
}
8. postman访问接口进行结果验证
#新建对象<br />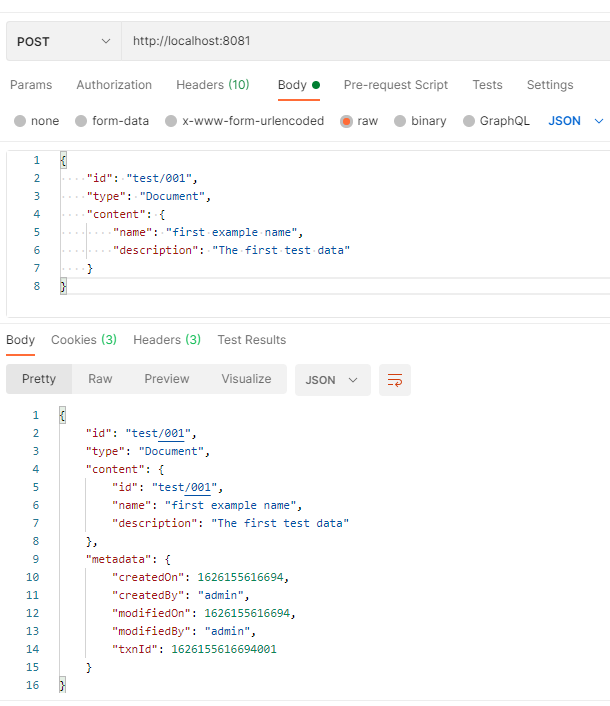<br />#获取单个对象<br />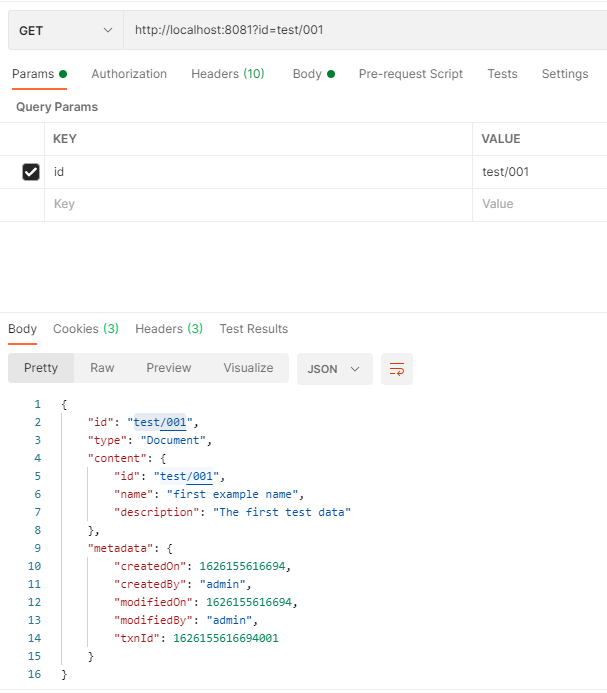<br />#更新对象<br />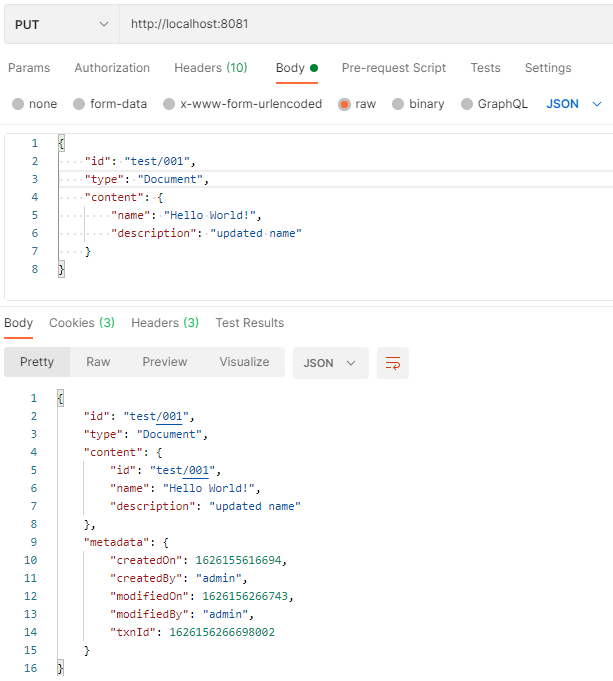<br />#删除对象<br />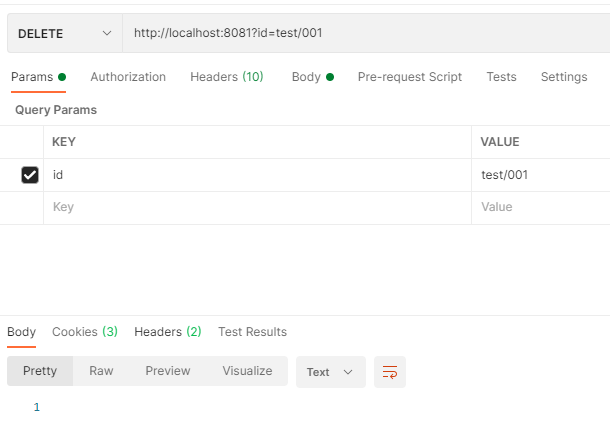<br />#获取所有对象http://localhost:8081/all,结果包含几个建立的系统数据对象,【id=test/123】的是另一个测试数据
```json
[
{
"id": "86.1234.abc.TEST/0cbd3f8ad53a8f1d8209",
"type": "Schema",
"content": {
"identifier": "86.1234.abc.TEST/0cbd3f8ad53a8f1d8209",
"name": "Document",
"schema": {
"type": "object",
"title": "Document",
"required": [
"id"
],
"properties": {
"id": {
"type": "string",
"cordra": {
"type": {
"autoGeneratedField": "handle"
}
}
},
"name": {
"type": "string",
"title": "Name",
"cordra": {
"preview": {
"showInPreview": true,
"isPrimary": true
}
}
},
"description": {
"type": "string",
"format": "textarea",
"title": "Description",
"cordra": {
"preview": {
"showInPreview": true
}
}
},
"creationDate": {
"type": "string",
"title": "Creation Date",
"cordra": {
"type": {
"autoGeneratedField": "creationDate"
}
}
},
"modificationDate": {
"type": "string",
"title": "Modification Date",
"cordra": {
"preview": {
"showInPreview": true
},
"type": {
"autoGeneratedField": "modificationDate"
}
}
},
"createdBy": {
"type": "string",
"title": "Created By",
"cordra": {
"type": {
"autoGeneratedField": "createdBy"
}
}
},
"modifiedBy": {
"type": "string",
"title": "Modified By",
"cordra": {
"type": {
"autoGeneratedField": "modifiedBy"
}
}
}
}
}
},
"metadata": {
"createdOn": 1626142554573,
"createdBy": "admin",
"modifiedOn": 1626142554573,
"modifiedBy": "admin",
"txnId": 1626142554541005
}
},
{
"id": "86.1234.abc.TEST/fe13890f0aed2e634da6",
"type": "Schema",
"content": {
"identifier": "86.1234.abc.TEST/fe13890f0aed2e634da6",
"name": "User",
"schema": {
"type": "object",
"required": [
"id",
"username",
"password"
],
"properties": {
"id": {
"type": "string",
"cordra": {
"type": {
"autoGeneratedField": "handle"
}
}
},
"username": {
"type": "string",
"title": "Username",
"cordra": {
"preview": {
"showInPreview": true,
"isPrimary": true
},
"auth": "username"
}
},
"password": {
"type": "string",
"format": "password",
"title": "Password",
"cordra": {
"auth": "password"
}
},
"requirePasswordChange": {
"type": "boolean",
"title": "Require Password Change",
"description": "If true a new password must be set on next authentication.",
"cordra": {
"auth": "requirePasswordChange"
}
}
}
},
"javascript": "exports.beforeSchemaValidation = beforeSchemaValidation;\n\nfunction beforeSchemaValidation(obj, context) {\n if (!context.useLegacyContentOnlyJavaScriptHooks) {\n obj.content = beforeSchemaValidationLegacy(obj.content, context);\n return obj;\n } else {\n return beforeSchemaValidationLegacy(obj, context);\n }\n}\n\nfunction beforeSchemaValidationLegacy(obj, context) {\n if (!obj.id) obj.id = \"\";\n if (!obj.password) obj.password = \"\";\n var password = obj.password;\n if (context.isNew || password) {\n if (password.length < 8) {\n throw \"Password is too short. Min length 8 characters\";\n }\n }\n return obj;\n}\n"
},
"metadata": {
"createdOn": 1626142554295,
"createdBy": "admin",
"modifiedOn": 1626142554295,
"modifiedBy": "admin",
"txnId": 1626142554262003
}
},
{
"id": "86.1234.abc.TEST/fec9da465d2c680caf42",
"type": "Schema",
"content": {
"identifier": "86.1234.abc.TEST/fec9da465d2c680caf42",
"name": "Group",
"schema": {
"type": "object",
"required": [
"id",
"groupName",
"description"
],
"properties": {
"id": {
"type": "string",
"cordra": {
"type": {
"autoGeneratedField": "handle"
}
}
},
"groupName": {
"type": "string",
"title": "Group Name",
"cordra": {
"preview": {
"showInPreview": true,
"isPrimary": true
}
}
},
"description": {
"type": "string",
"format": "textarea",
"maxLength": 2048,
"title": "Description",
"cordra": {
"preview": {
"showInPreview": true,
"excludeTitle": true
}
}
},
"users": {
"type": "array",
"format": "table",
"title": "Users",
"uniqueItems": true,
"items": {
"type": "string",
"title": "User",
"cordra": {
"type": {
"handleReference": {
"types": [
"User",
"Group"
]
}
}
}
},
"cordra": {
"auth": "usersList"
}
}
}
}
},
"metadata": {
"createdOn": 1626142554462,
"createdBy": "admin",
"modifiedOn": 1626142554462,
"modifiedBy": "admin",
"txnId": 1626142554429004
}
},
{
"id": "test/123",
"type": "Document",
"content": {
"id": "test/123",
"name": "first example name",
"description": "The first test data"
},
"metadata": {
"createdOn": 1626154301602,
"createdBy": "admin",
"modifiedOn": 1626154301602,
"modifiedBy": "admin",
"txnId": 1626154301607000
}
}
]