这篇笔记参考自https://www.imooc.com/video/12514。
资源
- 非官方的Laravel框架实战指南,长期更新,更易读好上手:Laravel 从入门到精通教程
- 小项目,小试牛刀的练手项目:博客系列教程 - 项目
- Laravel5.6 + Vu2的项目:基于 Laravel + Vue 构建前后端分离应用
路由
路由其实就是将用户的请求指派给相应的控制器进行处理,说白了就是建立URL与程序之间的映射关系。
在Laravel5.2中,路由定义在routes.php内。
基础路由
Route::get('/', function () {
return view('welcome');
});
多请求路由 - match:注册一个可响应多个 HTTP 动作的路由
Route::match(['get', 'post'], 'multiroute', function () {
return 'Hello World';
})
多请求路由 - any
Route::any('multiroute', function () {
return 'Hello World';
})
带参数路由:可以通过闭包的参数来处理参数,也可以使用where在尾部用正则表达式限制路由地址的参数类型
Route::get('user/{id}/{name?}', function ($id, $name = 'gaohang') {
return 'User name -' . $name . '- id' . $id;
}) -> where(['name' => '[A-Za-z]+', 'id' => '[0-9]+']);
路由别名:别名的作用在于如果我们修改路由地址,那么在controller中的调用的别名名字都不会改变,始终会把正确的URL显示出来。如下,
route('center')
就指代了public/user/lalala
这个路由! ```php Route::get(‘user/lalala’, [‘as’ => ‘center’, function () { // 给路由命名为center return route(‘center’); }]);
// http://localhost/laravel52/public/member/user/lalala
具体有什么用处呢?指定了路由名称,我们通过route('名称')即可返回真实的url,我们可以在视图中这样:
```html
// 链接
<a href="{{route('profile')}}">用户资料</a>
// 重定向
return redirect()->route('profile');
return redirect(route('profile'));
- 为路由指定处理控制器 ```php Route::get(‘user/profile’, [ ‘as’ => ‘profile’, ‘uses’ => ‘UserController@showProfile’ ]);
// 除了可以在路由的数组定义中指定路由名称外,你也可以在路由定义后方链式调用 name 方法: // name方法,为这条路由分配了名称 Route::get(‘user/profile’, ‘UserController@showProfile’)->name(‘profile’);
- 对命名路由生成对应Url:一旦你在指定的路由中分配了名称,则可通过 `route` 函数来使用路由名称生成 URLs 或重定向:
```php
$url = route('profile');
$redirect = redirect()->route('profile');
如果路由定义了参数,那么你可以把参数作为第二个参数传递给 route
方法。指定的参数将自动加入到 URL 中:
Route::get('user/{id}/profile', ['as' => 'profile', function ($id) {
//
}]);
$url = route('profile', ['id' => 1]); //得到http://xxx/user/1/profile 这样的url
也就是在后面传一个数组进去即可,数组的键名和路由参数名称对应。
路由群组 - group:为群组中的路由增加前缀,也就是member/user/lalala和member/multiroute2才能匹配到路由
Route::group(['prefix' => 'member'], function () {
Route::get('user/lalala', ['as' => 'center', function () {
return route('center');
}]);
Route::any('multiroute2', function () {
return 'Hello World 2';
});
});
路由中输出视图
Route::get('/', function () {
return view('welcome');
});
控制器
// routes.php
Route::get('member/info', 'MemberController@info'); 调用controller目录下的MemberController的info方法
// 同等写法
Route::get('member/info', ['uses' => 'MemberController@info'])
// 增加路由别名
Route::get('member/info', ['uses' => 'MemberController@info', 'as' => 'memberinfo'])
路由的参数可以传入controller对应的方法中,加限制可以在路由后调用where,传入限制的参数名与正则规则。
Route::any('member/{id}', ['uses' => 'MemberController@info'])
-> where('id', '[0-9]+');
request
在控制器中如何操作request参数(查询字符串),控制器方法接收Request $request
参数,相关的api如下:
- url() // 获取当前的完整url
- input(‘key’, ‘默认输出’)
- has(‘key’)
- all() // 返回所有ky对,也就是关联数组
- method() // 返回请求的类型
- isMethod(‘GET’)
- ajax() // 判断是否是ajax请求
- is(‘student/*’) // 判断路由格式,是否是student/下的一个请求路径
视图
return view('xxx')
,xxx为模板的名称。 ``` <?php
App\http\Controllers’;
class MemberController extends Controller { // 此时会返回resources下的member-info.php视图文件 public function info($id) { return view(‘member/memberInfo’, [ ‘name’ => ‘gaogao’, ‘age’ => 25, ‘gender’ => ‘male’ ]); } }
一般情况下,一个控制器对应views下一个目录,目录下存放对应的模板。同时,view方法后面可接收变量数组,在模板中通过`{{$name}}`\`{{$age}}`\`{{$gender}}`来调用。
<a name="U6j0D"></a>
## 模型
模型文件存放在app目录下,默认会新建一个User.php模型。模型在Controller中被调用,在controller中`use App\Member;`后,直接可以用调用Model中的方法,如`Member::getMember();`
<a name="fbW6Y"></a>
## 数据库操作
Laravel中提供了三种操作数据库的方式:
1. DB facade
1. 查询构造器
1. Eloquent ORM等
<a name="PRg8A"></a>
### 连接数据库
涉及两个文件的操作:config/databases.php和.env,<br />在控制器中,使用DB::SELECT('select * from ...'),use illuminate\Support\Facades\DB
<a name="ykSDu"></a>
### 1. DB Facade
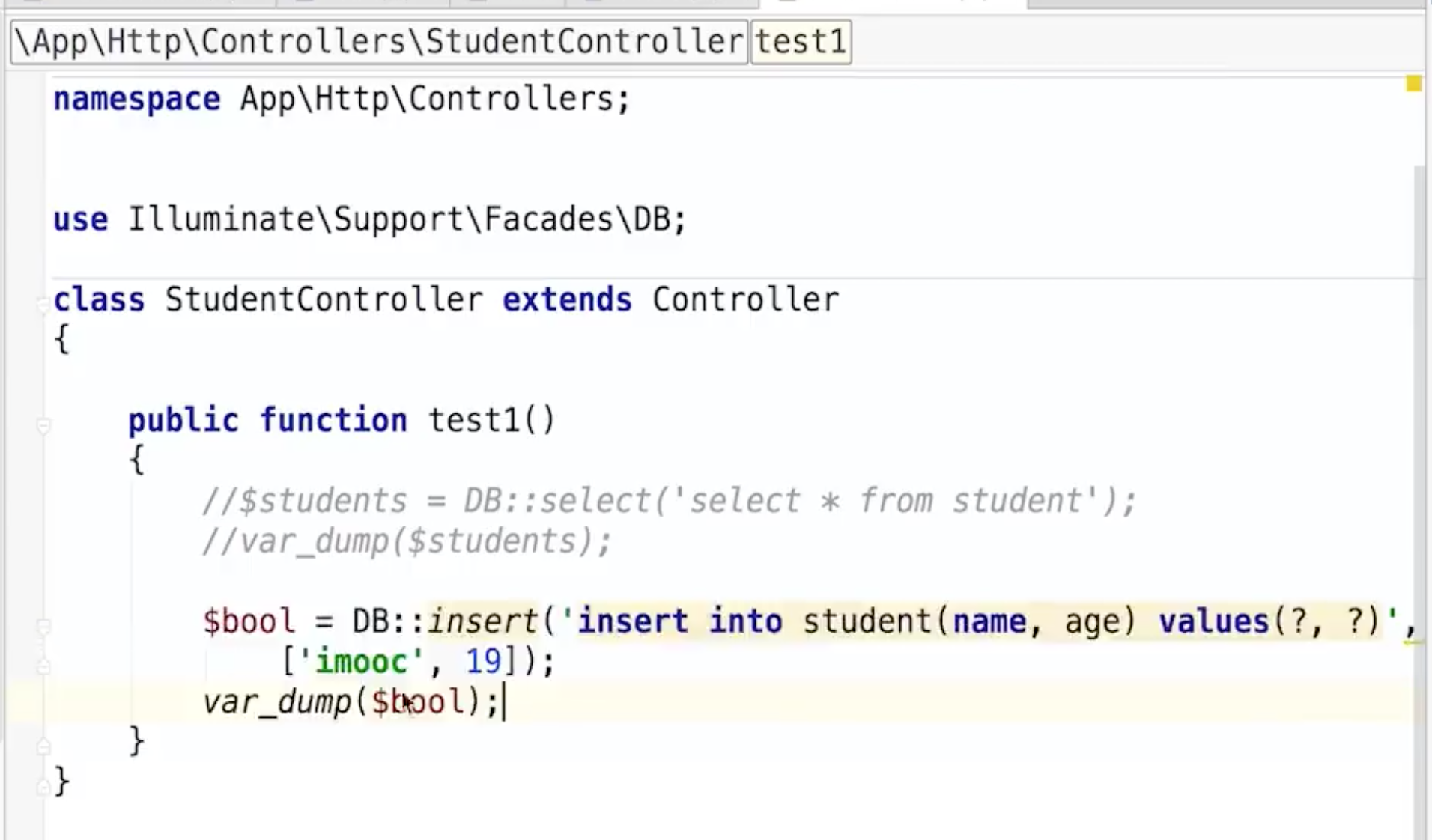<br />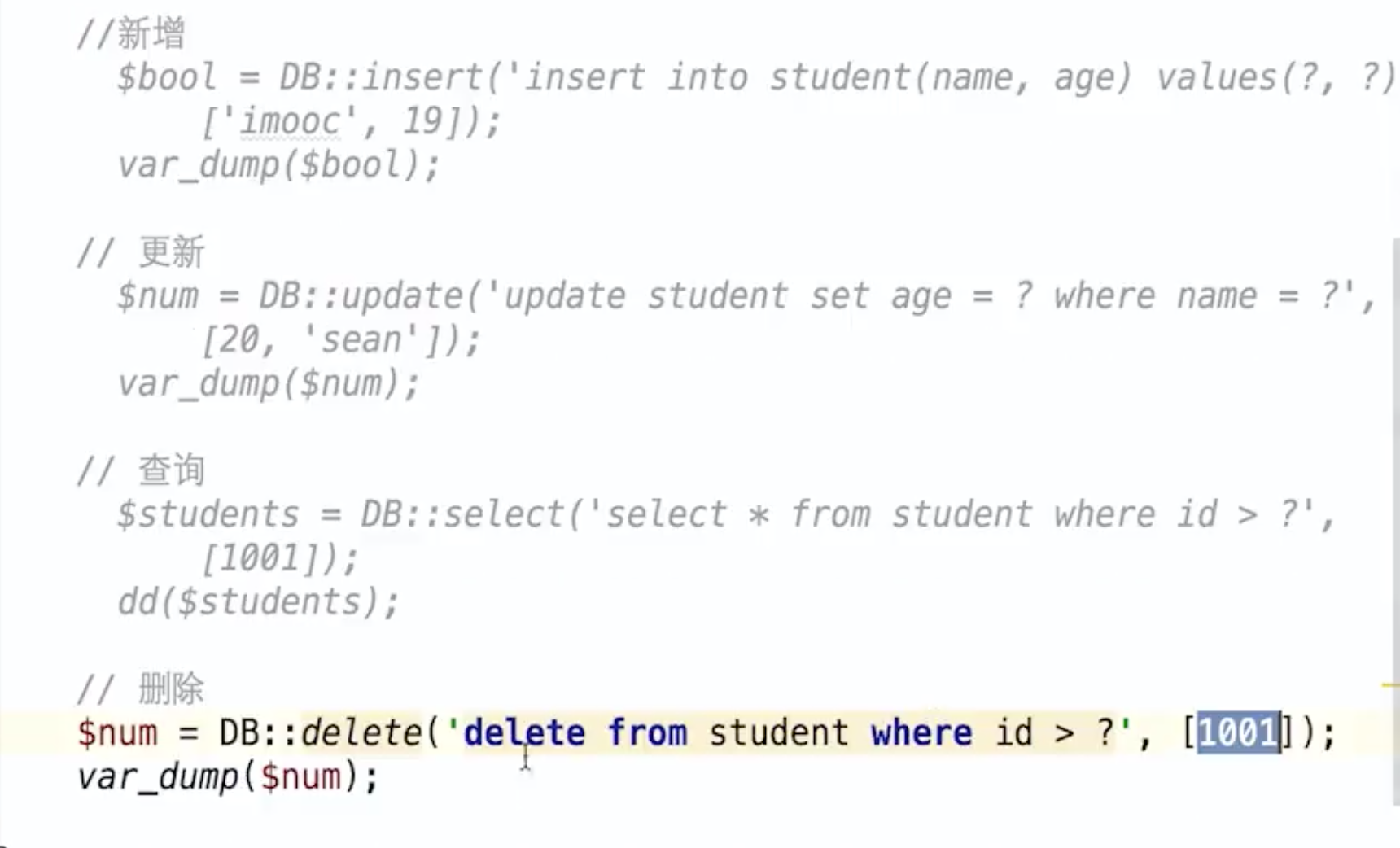
<a name="QMS7E"></a>
### 2. 查询构造器
- 插入数据
<?php // StudentController.php // 插入单条数据
App\http\Controllers’;
class StudentController extends Controller { public function query() { $bool = DB::table(‘student’)->insert( // 返回插入结果 [‘name’ => ‘imooc’, ‘age’ => 18] );
DB::table('student')->insertGetId(
['name' => 'imooc', 'age' => 18] // 返回自增Id
);
DB::table('student')->insert([ // 插入多条数据 多维数组
['name' => 'imooc', 'age' => 18],
['name' => 'hang', 'age' => 19],
]);
} };
- 更新数据
```php
public function update()
{
$line = DB::table('student')
->where('id', 1004) // 更复杂的条件 where('id','>=',5)
->update(['age' => 18]);
var_dump($line);
$num1 = DB::table('student')
->increment('age'); // 所有自动加1
$num2 = DB::table('student')
->increment('age', 3); // 所有自动加3
$num3 = DB::table('student')
->where('id', 1002)
->decrement('age', 3);
}
删除数据
public function delete()
{
$line = DB::table('student')
->where('id', '>=', '1004')
->delete();
var_dump($line);
}
查询数据
用到的api有:get(), first(), where(), pluck(), lists(), select(), chunk()
- get():返回整个表的数据;
first():返回一条记录,默认随机获取第一条
$students = DB::table('student')
->whereRaw('id >= ? and age > ?', [1001, 18]) // 指定多个条件的方法
->get();
dd($students);
pluck():返回结果集中指定的字段(表字段)
- lists():同上,但可以同时指定value ``` $students = DB::table(‘student’) ->pluck(‘name’); dd($students); // 返回name为value的索引数组
$students = DB::table(‘student’) ->lists(‘name’, ‘id’); dd($students); // 返回id为key,name为value的关联数组
- select():指定需要查询的字段,传入需要的字段名,逗号隔开,相当于get的key筛选
- chunk():对数据分段获取,第一个参数的每次的查询数量,第二个参数是闭包,可根据条件用`return false`来中断查询;
- 聚合函数
- count():返回表的记录数
- max():传入字段名,返回最大值
- min()
- avg()
- sum()
<a name="HowlX"></a>
### 3. Eloquent ORM
Laravel自带的Eloquent ORM是ActiveRecord实现,用来进行数据库交互。最大特点就是每个table都有一个与之相对应的Model文件用来交互!
- all():返回一个集合,items键的值是结果数组,attributes为表的字段
- find():传入主键,查找单条集合
- findOrFail():根据指定查找,查找不到则报错;
- Student::where('id', '>=', '1001')->orderBy('age', 'desc')->first():同样也可以获取第一条
- Student::chunk(2, function($students) { var_dump($students) });
- Student::where('id', '>', 1001)->max('age');:聚合函数一样可以用
<a name="YHbiU"></a>
### 新增数据:使用模型
// app/Models/Student.php // 可控制新增数据时timestamp是否自动维护 protected $timestamps = true;
// 默认返回当前时间 protected function getDateFormat() { return time(); }
// 取消默认的事件处理 自己进行格式化即可 protected function asDateTime($value) { return $value; }
// StudentController.php public function orm() { // $students = Student::all(); // dd($students); $student = new Student(); $student->age = 25; $student->name = ‘gaogao’; $bool = Student->save(); // 保存数据 dd($student);
$student = $student::find(1002);
echo date('Y-m-d H:i:s', $student->created_at);
}
<a name="FCPJD"></a>
### 新增数据:使用模型的create方法
// Model中指定允许批量赋值的字段
// 指定允许批量赋值的字段 protected $fillable = [‘name’, ‘age’];
// 不允许批量赋值的字段 protected $guarded = [‘created_at’];
// StudentController.php public function orm() { $student = Student::create( // 使用create方法创建数据 [‘name’ => ‘gaogao’, ‘age’ => 18] ); dd($student);
// firstOrCreate(['name' => 'high']) 以属性查找,如果没有则新建
// firstOrNew() 以属性查找 没有则新建新的实例 需要保存则自己再调用save方法
$student = Student::firstOrNew(
['name' => 'hanghang']
);
$bool = $student->save();
dd($bool);
}
<a name="ZGnad"></a>
### 更新数据:使用模型
public function orm() { $student = Student::find(1014); $student->name = ‘new Name’; $bool = $student->save(); var_dump($bool); // true }
<a name="LETBc"></a>
### 更新数据:结合查询语句批量更新
public function orm() { $number = Student::where(‘id’, ‘>’, 1019)->update( [‘age’ => 41] ) }
<a name="nLin2"></a>
### 删除数据:模型删除
public function orm() { $student = Student::find(1014); $bool = $student->delete(); }
<a name="IYsAu"></a>
### 删除数据:primarykey删除
public function orm() { $bool = Student::destroy([1016, 1017]); }
<a name="ZGlXR"></a>
### 删除数据:条件删除
public function orm() { $number = Student::where(‘id’, ‘>’, 1019)->delete(); } ```