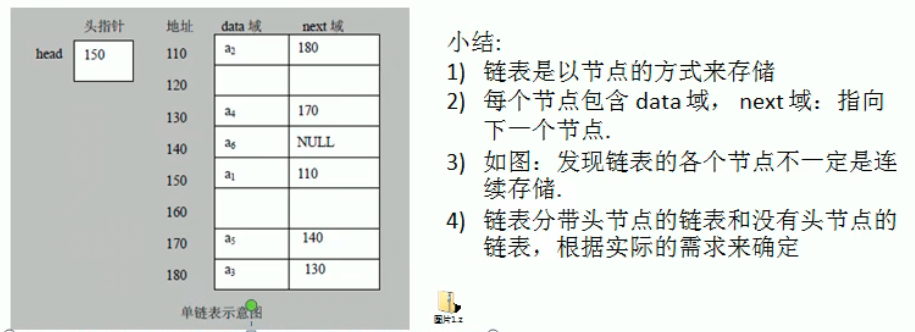
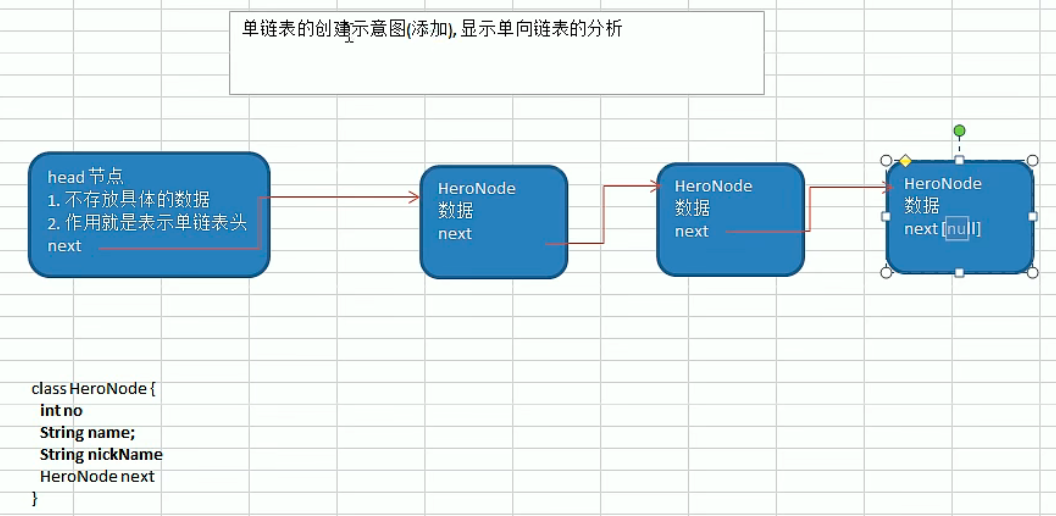
@Data
@NoArgsConstructor
@AllArgsConstructor
@ToString
public class HeroNode {
private int no;//排名
private String name; //名称
private String nickname; //别名
private HeroNode next; //指向下一个节点
public HeroNode(int no, String name, String nickname) {
this.no = no;
this.name = name;
this.nickname = nickname;
}
}
//单向链表
public class SingleLinkedList {
//创建头结点
private static HeroNode head = new HeroNode(1,"宋江","及时雨",null);
/**
* 有序添加; 退添加的节点进行排序
* @param newNode 被添加的节点
* @return
*/
public boolean addNodeOrder(HeroNode newNode){return addNodeOrderRecursion(head,newNode);}
private static boolean addNodeOrderRecursion(HeroNode node,HeroNode newNode){
if(node.getNext() != null){
//编号重复
if(node.getNo() == newNode.getNo()){
System.out.println("编号重复");
return false;
}
//排序
if(node.getNo()<newNode.getNo() && newNode.getNo()<node.getNext().getNo()){
newNode.setNext(node.getNext());
node.setNext(newNode);
}else{
addNodeOrderRecursion(node.getNext(),newNode);
}
}else{
node.setNext(newNode);
}
return true;
}
/**
* 不排序添加;疯狂往后面扔
* @param newNode 被添加的节点
* @return
*/
public boolean addNode(HeroNode newNode){return addNodeRecursion(head,newNode);}
private static boolean addNodeRecursion(HeroNode node,HeroNode newNode){
if(node.getNext() != null){
//编号重复
if(node.getNo() == newNode.getNo()){
System.out.println("编号重复");
return false;
}
addNodeRecursion(node.getNext(),newNode);
}else{
node.setNext(newNode);
}
return true;
}
/**
* 遍历所有节点
*/
public void nodeList(){nodeListRecursion(head);}
private void nodeListRecursion(HeroNode node){
System.out.print("排名: "+node.getNo());
System.out.print(" 名字:\t"+node.getName());
System.out.println(" 外号:\t"+node.getNickname());
if(node.getNext() != null){
nodeListRecursion(node.getNext());
}else{
return;
}
}
/**
* 根据 no 修改
* @param heroNode
* @return
*/
public boolean updateNode(HeroNode heroNode){ return updateNodeRecuresion(head,heroNode);}
private boolean updateNodeRecuresion(HeroNode node , HeroNode heroNode){
///假设当前的节点满足
if(node.getNo() == heroNode.getNo()){
node.setName(heroNode.getName());
node.setNickname(heroNode.getNickname());
System.out.println("修改成功");
return true;
}
if(node.getNext() != null){
return updateNodeRecuresion(node.getNext(),heroNode);
}else{
System.out.println("没有找到该节点");
return false;
}
}
/**
* 根据 no 删除
* @param no
* @return
*/
public boolean delteNode(int no){ return delteNodeRecuresion(head,no);}
private boolean delteNodeRecuresion(HeroNode node,int no){
if(node.getNext() !=null && node.getNext().getNo() == no){
node.setNext(node.getNext().getNext());
System.out.println("删除成功");
return true;
}
if(node.getNext() != null){
return delteNodeRecuresion(node.getNext(),no);
}else {
System.out.printf("未找到编号 %d 的节点",no);
System.out.println();
return false;
}
}
/**
* 通过 no 获取
* @param no
* @return
*/
public HeroNode selectNode(int no){ return selectNodeRecuresion(head,no);}
private HeroNode selectNodeRecuresion(HeroNode heroNode,int no){
if(heroNode.getNo() == no){
return heroNode;
}
if(heroNode.getNext() !=null){
return selectNodeRecuresion(heroNode.getNext(),no);
}else{
System.out.printf("为找到编号为 %s 的节点",no);
return null;
}
}
/**
* 获取倒数第n的节点
* @param index
* @return
*/
public HeroNode reciprocalNode(int index){if(index==0) index=1;return reciprocalNodeRecuresion(head,nodeCount()-index);}
private HeroNode reciprocalNodeRecuresion(HeroNode heroNode,int num){
if(num<0){return null;}
if(num != 0){
num--;
return reciprocalNodeRecuresion(heroNode.getNext(),num);
}else{
return heroNode;
}
}
/**
* 获取节点个数
* @return
*/
public int nodeCount(){ return nodeCountRecuresion(head,0);}
private int nodeCountRecuresion(HeroNode heroNode,int count){
if(heroNode.getNext() != null){
count++;
return nodeCountRecuresion(heroNode.getNext(),count);
}else{
count++;
return count;
}
}
/**
* 反转
* @return
*/
public HeroNode reversal(){reversalRecuresion(head);return head;}
private static void reversalRecuresion(HeroNode heroNode){
if (heroNode.getNext() != null){
reversalRecuresion(heroNode.getNext());
heroNode.setNext(null);
addNodeRecursion(head,heroNode);
}else{
head = heroNode;
return;
}
}
}
public class Test {
public static void main(String[] args) {
SingleLinkedList singleLinkedList = new SingleLinkedList();
HeroNode ljy = new HeroNode(2, "卢俊义", "玉麒麟");
HeroNode wy = new HeroNode(3, "吴用", "智多星");
HeroNode zs = new HeroNode(4, "张三", "路人甲");
HeroNode ls = new HeroNode(5, "李四", "路人乙");
HeroNode zl = new HeroNode(6, "赵六", "路人丙");
//添加
singleLinkedList.addNodeOrder(ljy);
singleLinkedList.addNodeOrder(ls);
singleLinkedList.addNodeOrder(wy);
singleLinkedList.addNodeOrder(zs);
singleLinkedList.addNodeOrder(zl);
//修改
HeroNode lbw= new HeroNode(2, "卢本伟", "伞兵一号");
singleLinkedList.updateNode(lbw);
//通过 no 删除
singleLinkedList.delteNode(5);
//倒叙
singleLinkedList.reversal();
//遍历
singleLinkedList.nodeList();
//通过编号查询
HeroNode selectNode = singleLinkedList.selectNode(2);
System.out.println("----------------");
System.out.printf("排名: %d\t名字: %s\t外号:%s",selectNode.getNo(),selectNode.getName(),selectNode.getNickname());
System.out.println();
//获取倒叙第 n 个节点
HeroNode reciprocalNode = singleLinkedList.reciprocalNode(0);
System.out.println("----------------");
System.out.printf("排名: %d\t名字: %s\t外号:%s",reciprocalNode.getNo(),reciprocalNode.getName(),reciprocalNode.getNickname());
System.out.println();
//获取节点个数
System.out.println(singleLinkedList.nodeCount());
}
}