简单CRUD
<?php
class Crud extends CI_Controller
{
public function __construct()
{
parent::__construct();
$this->load->database();
}
/*
* create
*/
public function c() {
$arrUser = array();
$arrUser['loginname'] = 'admin';
$arrUser['username'] = 'administrator';
$this->db->insert('cicms_users', $arrUser);
}
/*
* retrieve
*/
public function r() {
$arrParams = $this->uri->uri_to_assoc();
if (empty($arrParams) || empty($arrParams['id'])) {
echo 'no parameters';
return;
}
$this->db->from('cicms_users')->where('id=', $arrParams['id']);
$res = $this->db->get();
var_dump($res->result());
}
/*
* retrieve all
*/
public function ra() {
$res = $this->db->get('cicms_users');
var_dump($res->result());
}
/*
* updata
*/
public function u() {
$this->db->set('username', 'username111');
$this->db->where('id', 1);
var_dump($this->db->update('cicms_users'));
}
/*
* delete
*/
public function d() {
$this->db->where('id', 1);
var_dump($this->db->delete('cicms_users'));
}
}
Controlle从前端获取参数
class Ctrl extends CI_Controller
{
public function __contruct() {
parent::__contruct();
ECHO __METHOD__, '<br>';
}
public function index() {
echo __METHOD__;
}
public function params() {
var_dump($this->uri->uri_to_assoc());
}
}
入门案例
在application文件夹config目录下找到router文件,将其打开,修改index.php路由时指向自定义的控制器。
【例1】设计一个hello网站
Controllers目录中添加一个Hello控制器即Hello.php,并写入默认的index方法,装载视图为hello。
class Hello extends CI_Controller { public function index() { $this->load->view('hello'); } }
在Views目录中添加hello.php文件,在其中写入代码。 ```php <!DOCTYPE html>
<?php
echo 'helloworld';
?>
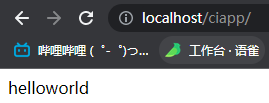
<a name="igaa3"></a>
#### 【例2】控制器方法传递参数
Hello类下新加test方法
```php
class Hello extends CI_Controller
{
public function index()
{
$this->load->view('hello');
}
public function test() {
// 定义两个变量
$data['age'] = 23;
$data['gender'] = 'male';
// 将变量数组$data传递到me视图
$this->load->view('hello', $data);
}
}
在views目录下hello.php修改
<!DOCTYPE html>
<html>
<head>
<title>hello网站示例</title>
</head>
<body>
<h3>自定义控制器方法传递变量示例</h3>
<?php echo '我的年龄'.$age; ?></body>br>
<?php echo '我的年龄'.$gender; ?></body>br>
</body>
</html>
【例3】视图页面文件之间的路由
在views目录下修改hello.php文件
<!DOCTYPE html>
<html>
<head>
<title>hello网站示例</title>
</head>
<body>
<h3>页面视图路由示例</h3>
<a href="<?php echo site_url('hello'); ?>">查看hello视图,测试site_url()函数</a>
<a href="<?php echo base_url('public/img/renhaoshuai.jpg'); ?>">查看图片,测试base_url()函数</a>
<?php echo '我的年龄'.$age; ?></body>br>
<?php echo '我的年龄'.$gender; ?></body>br>
</body>
</html>
控制器test方法添加$this->load->helper('url'); //装载helper辅助url方法
site():控制根目录路由 site(‘控制器/方法’)
base_url():网站根目录路由 base_url(‘根目录’)
【例4】GET方式取值
在Hello.php添加math方法
public function math() {
$a = $this->input->get('a'); //获取变量a的值
$b = $this->input->get('b'); //获取变量b的值
echo '加法的结果为:'.($a + $b);
}
【例5】POST方式发送值
修改hello.php视图和控制器
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<h3>hello网站示例</h3>
<form action="<?php echo site_url('Hello/login'); ?>" method="post">
<div>
<label for="username">用户名</label>
<input type="text" name="username">
</div>
<div>
<label for="userpwd">用户密码</label>
<input type="password" name="userpwd">
</div>
<div>
<input type="submit" name="submit" value="提交">
</div>
</form>>
</body>
</html>
public function login(){
$username = $this->input->post('username');
$userpwd = $this->input->post('userpwd');
echo '提交过来的用户名为:'.$username;
}
【例6】多个视图文件同时调用
在views文件夹下新建header.php和footer.php
<header>
<table width="600px" border="1" bgcolor="#999">
<tr>
<td>CI简介</td>
<td>CI性能</td>
<td>CI应用</td>
<td>CI扩展</td>
</tr>
</table>
</header>
<footer style="width: 600px;font-size: 14px;text-align: center;">
<span>copyright@2019-2025,联系方式1115189918@qq.com</span>
</footer>
在hello控制器里面,index方法中按顺序导入多个视图
class Hello extends CI_Controller
{
public function index()
{
$this->load->helper('url');
$this->load->view('header');
$this->load->view('hello');
$this->load->view('footer');
}
public function welcome() {
$this->load->view('header');
$this->load->view('welecome_message');
$this->load->view('footer');
}
再将地址换成
【例7】在hello网站查数据库显示
- 在models文件夹下新建User_Model.php文件
```php
<?php
class User_Model extends CI_Controller{
//构造函数里面连接好数据库
function __construct()
{
} public function userList(){parent::__construct(); $this->load->database();
} }$res = $this->db->get('user')->result_array(); //读取user表中数据 return $res; //将读取的数据返回传递到控制器
2. 在控制器中新建User.php
```php
<?php
class User extends CI_Controller{
public function userinfo(){
$this->load->model('User_Model');
$data['userinfo']=$this->User_Model->userList();
$this->load->view('userpage', $data);
}
}
- 在views目录下新建userpage.php文件
<table border="1"> <tr> <td>用户名</td> <td>用户密码</td> <td>用户注册时间</td> </tr> <?php foreach ($userinfo as $key => $value) {?> <tr> <td><?php echo $value['username']; ?></td> <td><?php echo $value['userpwd']; ?></td> <td><?php echo $value['reg_time']; ?></td> </tr> <?php } ?> </table>
缓存与日志
【例8】设置页面缓存
控制器index方法中添加,然后访问http://localhost/ciapp/index.php/,application的cache目录下就会多出一个文件
$this->output->cache(50); //设置页面缓存时间为50m
$this->output->delete_cache('/foo/bar'); //删除缓存
配置文件中也可配置数据库缓存
【例9】网页运行日志
默认不开启日志,开启在application目录下的config下的config.php将其设置为
$config['log_threshold'] = 0;
0:表示不开启日志;1表示开始记录错误日志;2表示开启调试日志;3表示信息日志;4表示所有信息。
$config['log_path'] = ''; //设置保存日志路径,默认aplication/logs下
$config['log_file_extension'] = ''; //设置后缀名,默认为.php后缀
CodeIgniter类库
在system文件夹libraries目录下,就是CI框架自带的类,可以参考用户手册。
Pagination分页类
修改models目录下User_Model.php模型文件
<?php class User_Model extends CI_Controller{ //构造函数里面连接好数据库 function __construct() { parent::__construct(); $this->load->database(); } public function userList($per_page){ //获取URL上第4段的数,确定为当前页起始数 $currpage = $this->uri->segment(4) == '' ? 0 : $this->uri->segment(4); //查询总记录数 $total_rows = $this->db->query('select count(userID) as total from user')->row_array(); //从user表里根据给定limit范围查询数据 $curr_res = $this->db->query("select * from user order by userID asc limit {$currpage},{$per_page}")->result_array(); //总记录数保存在data数组里 $data['total'] = $total_rows['total']; //每一页的记录存在data数组里 $data['data'] = $curr_res; return $data; } }
User控制器文件加入分页标记
<?php class User extends CI_Controller // 新建控制器类名 User, 继承 CI 控制器 { public function userinfo(){ // 新建 userinfo 方法 $this->load->library('pagination'); // 导入分页类库 $this->load->helper('url'); // 导入辅助类 url $this->load->model('User_Model'); // 导入模型文件 $per_page = 4; // 每页显示 4 个记录 $config['base_url'] = site_url('User/userinfo/page'); // 给定 url 根地址 $config['per_page'] = $per_page; $config['reuse_query_string'] = true; $config['first_link'] = false; $config['prev_link'] = '«'; $config['next_link'] = '»'; $config['last_link'] = false; $config['next_tag_open'] = '<li>'; $config['next_tag_close'] = '</li>'; $config['num_tag_open'] = '<li>'; $config['num_tag_close'] = '</li>'; $config['prev_tag_open'] = '<li>'; $config['prev_tag_close'] = '</li>'; $config['cur_tag_open'] = '<li class="active"><a href="#">'; $config['cur_tag_close'] = '</a></li>'; $rs = $this->User_Model->userList($per_page); // 获取每页的记录数据 $config['total_rows'] = $rs['total']; $data['users'] = $rs['data']; $this->pagination->initialize($config); $data['page']=$this->pagination->create_links(); $this->load->view('userpage',$data); } }
试图文件userpage增加一个显示分页标记
session会话类
后盾网
项目搭建
application\config\autoload.php配置自动加载
$autoload['helper'] = array('url');
application\controllers和application\views新建两个文件夹一个前台index和一个后台admin,index下新建默认控制器home.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* 默认前台控制器
*/
class Home extends CI_Controller {
/**
* 默认首页显示方法
*/
public function index() {
echo 'hello';
}
}
配置默认控制器,找到路由application\config\routes.php修改
$route['default_controller'] = 'index/home';
浏览器访问http://localhost/ci_article/index.php/index/Home/index页面显示出hello
复制模板文件home.html和category.html和article.html到view/index文件夹中,更改Home.php再次访问
public function index() {
$this->load->view('index/home.html');
}
根目录新建样式文件夹style下再新建admin和index,引入样式文件后,更改controller访问测试
http://localhost/ci_article/index.php/index/Home/index
注:无模板文件只有源码,我只复制了源码但是会报错,先跟着做
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* 默认前台控制器
*/
class Home extends CI_Controller {
/**
* 默认首页显示方法
*/
public function index() {
$this->load->view('index/home.html');
}
/**
* 分类页显示方法
*/
public function category() {
$this->load->view('index/category.html');
}
/**
* 文章阅读页显示方法
*/
public function article() {
$this->load->view('index/article.html');
}
}
controllers下admin下新建后台默认控制器,访问测试http://localhost/ci_article/index.php/admin/admin/index
/**
* 后台默认控制器
*/
class Admin extends CI_Controller {
/**
* 默认方法
*/
public function index(){
$this->load->view('admin/index.html');
}
/**
* 默认欢迎
*/
public function copy(){
$this->load->view('admin/copy.html');
}
}
application\views\admin\index.html更改
后台登陆控制器application\controllers\admin\Login.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* 后台默认控制器
*/
class Login extends CI_Controller {
/**
* 默认方法
*/
public function index(){
$this->load->view('admin/login.html');
}
}
表单验证
application\controllers\admin新建文章管理控制器Article.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* 后台文章管理控制器
*/
class Article extends CI_Controller {
/**
* 发表模板显示
*/
public function send_article(){
$this->load->view('admin/article.html');
}
/**
* 发表文章动作 检查填入信息
*/
public function send(){
$this->load->library('form_validation');
$this->form_validation->set_rules('title', '文章标题', 'required|min_length[5]');
$status = $this->form_validation->run(); //成功返回true失败false
var_dump($status);
}
}
application\language引入汉化包ch,config.php修改English为ch
$config['language'] = 'ch';
表单验证配置application\config\form_validation.php
<?php
$config = array(
'article' => array(
array(
'field' => 'title',
'label' => '标题',
'rules' => 'required|min_length[5]'
),
array(
'field' => 'type',
'label' => '类型',
'rules' => 'required|integer'
),
array(
'field' => 'cid',
'label' => '栏目',
'rules' => 'integer'
),
array(
'field' => 'info',
'label' => '摘要',
'rules' => 'required|max_length[155]'
),
array(
'field' => 'content',
'label' => '内容',
'rules' => 'required|max_length[2000]'
)
),
'cate' => array(
array(
'field' => 'cname',
'label' => '栏目名称',
'rules' => 'required|max_length[20]'
),
),
);
修改Article.php一行搞定
$status = $this->form_validation->run('article'); //成功返回true失败false
编辑文章application\controllers\admin\Article.php
/**
* 编辑文章
*/
public function edit_article(){
$this->load->helper('form');
$this->load->view('admin/edit_article.html');
}
/**
* 编辑动作
*/
public function edit(){
$this->load->library('form_validation');
$status = $this->form_validation->run('article');
if($status){
echo '数据库操作';
} else {
$this->load->helper('form');
$this->load->view('admin/edit_article.html');
}
}
添加栏目
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Category extends CI_Controller {
/**
* 添加栏目
*/
public function add_cate() {
$this->load->helper('form'); //载入模板
$this->load->view('admin/add_cate.html');
}
/**
* 添加动作
*/
public function add() {
$this->load->library('form_validation');
$status = $this->form_validation->run('cate');
if($status){
echo '数据库操作';
} else {
$this->load->helper('form');
$this->load->view('admin/add_cate.html');
}
}
}
操作数据库
application\controllers\admin\category.php
/**
* 添加动作
*/
public function add() {
$this->load->library('form_validation');
$status = $this->form_validation->run('cate');
if($status){
$data = array(
'cname' => $this->input->post('cname');
);
$this->load->model('Category_model', 'cate');
$this->cate->add($data);
succes('admin/category/index', '添加成功');
} else {
$this->load->helper('form');
$this->load->view('admin/add_cate.html');
}
}
新建application\models\Category_model.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* 栏目管理模型
*/
class Category_model extends CI_Model {
/**
* 添加
*/
public function add($data) {
$this->db->insert('category', $data);
}
}
更改application\controllers\admin\category.php
创建数据库表
CREATE TABLE `hd_category` (
`cid` int(11) NOT NULL AUTO_INCREMENT,
`cname` varchar(15) COLLATE utf8_unicode_ci NOT NULL,
PRIMARY KEY (`cid`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci
$autoload['libraries'] = array('database');
$db['default'] = array(
'dsn' => '',
'hostname' => 'localhost',
'username' => 'root',
'password' => 'root',
'database' => 'ci_article',
'dbdriver' => 'mysqli',
'dbprefix' => 'hd_',
更改application\config\config.php为true,get和post做跨站处理
$config['global_xss_filtering'] = TRUE;
system\core\Common.php下定义公共用函数
/**
* 下面是自定义函数
*/
function p($arr) {
echo '<pre>';
print_r($arr);
echo '</pre>';
}
/**
* 成功提示函数
*/
function success($url, $msg) {
header('Content-Type:text/html;charset=utf-8');
$url = site_url($url);
echo "<script type='text/javascript'>alert('$msg');location.href='$url'</script>";
die;
}
/**
* 错误提示函数
*/
function error($msg) {
header('Content-Type:text/html;charset=utf-8');
echo "<script type='text/javascript'>alert('$msg');window.history.back();</script>";
die;
}
栏目管理模型Category_model新建
/**
* 查看栏目
*/
public function check() {
$data = $this->db->get('category')->result_array();
p($data);
}
class Category extends CI_Controller {
public function index() {
$this->load->model('category_model', 'cate');
$data['category'] = $this->cate->check();
$this->load->view('admin/cate.html');
}
根据ID编辑栏目application\models\category_model.php
/**
* 根据id查询栏目
*/
public function check_cate($cid) {
//where最好传数组,帮助过滤安全处理
$data = $this->db->where(array('cid'=>$cid))->get('category')->result_array();
return $data;
}
application\controllers\admin\category.php
/**
* 编辑栏目
*/
public function edit_cate(){
$cid = $this->uri->segment(4);
$this->load->model('category_model', 'cate');
$data['category'] = $this->cate->check_cate($cid);
$this->load->helper('form');
$this->load->view('admin/edit_cate.html', $data);
}
application\views\admin\edit_cate.html
<body>
<form action="<?php echo site_url('admin/category/edit') ?>" method="POST">
<table class="table">
<tr>
<td class="th" colspan="10">编辑栏目</td>
</tr>
<tr>
<td>栏目名称</td>
<td><input type="text" name="cname" value="<?php echo $category[0]['cname'] ?>"/><?php echo form_error('cname', '<span>', '</span>') ?></td>
</tr>
<tr>
<input type="hidden" name="cid" value="<?php echo $category[0]['cid'] ?>"/>
<td colspan="10"><input type="submit" value="编辑" class="input_button"/></td>
</tr>
</table>
</form>
</body>
编辑完之后需要提交